How to Merge a Specific Commit in Git
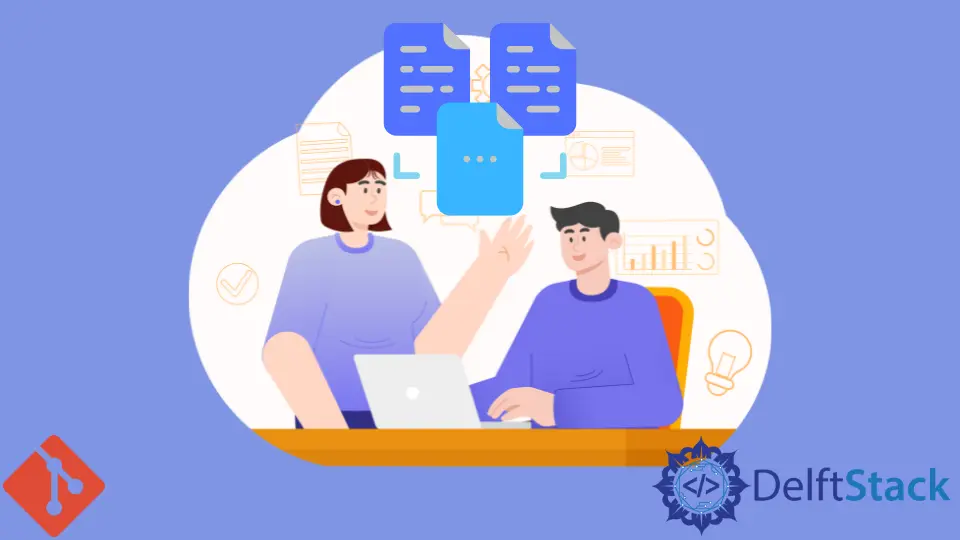
This article will discuss how we can merge a specific commit in Git. This is handy when we want to move a commit of our choosing to a different branch while working on a project.
Let’s jump right in.
Merge a Specific Commit in Git
Below are the four steps we use when merging a specific commit in Git.
-
Fetch Changes from Remote Repository
We use the
git fetch
command to download any changes made to the remote repository to our local machine.git fetch
Note that the above command imports only the changes and stores them in your local repo. It does not merge the commits.
-
Confirm the Commit Hash
You will need the commit hash of the commit you want to merge. Follow these steps.
Switch to the branch containing the commit you desire.
git checkout <branch-name>
Run the
git log
command to see a list of the commits in that branch. Use the--oneline
argument for a compact view.git log --oneline
-
Merge the Commit
Note down the hash of the commit you want to merge and switch to the destination branch. Use the
git checkout
command.git checkout <branch-name>
Use the
git cherry-pick
command to merge the commit you desire with your current branch.git cherry-pick <sha1-commit-hash>
-
Push the Branch
We can now run the
git push
command to push the changes to the remote repository.git push origin <branch-name>
It is worth noting that we use the
git merge
command to combine two Git branches. We can also use the command to merge multiple commits to one history.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn