How to List Remote Branches in Git
Stewart Nguyen
Feb 02, 2024
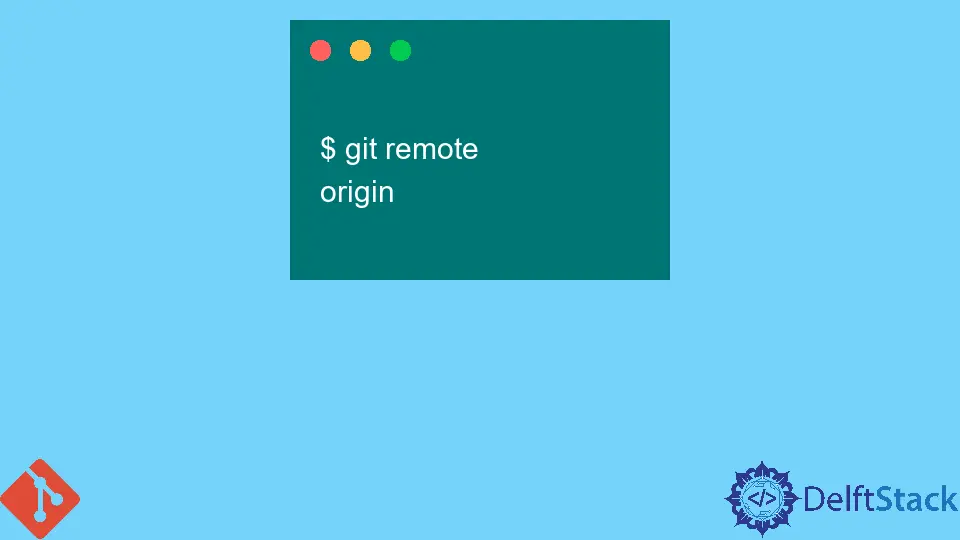
This article will introduce how to list remote repositories from your local branch.
Remote repositories are projects hosted on the server, such as Github/Gitlab.
git remote
allows us to use a short name (alias) to execute commands instead of typing a whole remote URL.
$ git fetch git@github.com:stwarts/git-demo.git
From github.com:stwarts/git-demo
* branch HEAD -> FETCH_HEAD
Use git remote -v
for listing detail of all remotes, to display just the names, use git remote
.
The -v
option stands for --verbose
, which shows the corresponding URL to each name.
$ git remote
origin
$ git remote -v
origin git@github.com:stwarts/git-demo.git (fetch)
origin git@github.com:stwarts/git-demo.git (push)
Related Article - Git Remote
- How to Add SSH in Git Remote
- How to Remove Upstream Repository in Git
- How to Create a Remote Repository From a Local Repository in Git
- How to Synchronize a Local Repository With a Remote Repository in Git
- How to Update a Repository by Setting Up a Remote
- How to Push From an Existing Remote Repository to a Different Remote Repository in Git