How to Ignore Local File Changes in Git
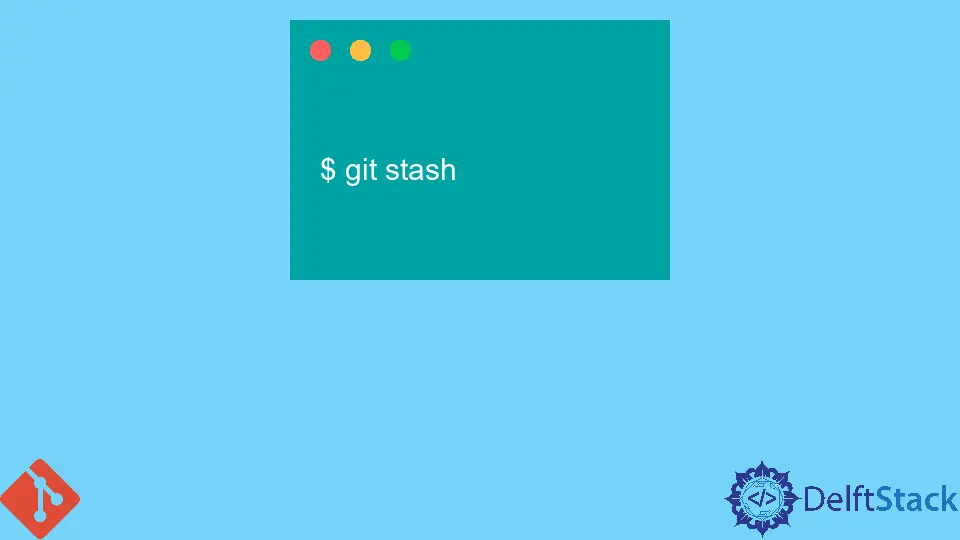
Managing local changes in Git can sometimes feel overwhelming, especially when you’re trying to sync with a remote repository. You may have uncommitted changes that you don’t want to lose or interfere with an update from the remote. Fortunately, Git provides several methods to ignore local file changes temporarily, allowing you to pull updates without affecting your work.
In this article, we’ll explore effective techniques to ignore local file changes in Git, ensuring you can keep your workflow smooth and efficient. Whether you’re a seasoned developer or just starting, understanding these methods will enhance your version control skills and help you manage your codebase more effectively.
Using Git Stash
One of the most common ways to ignore local changes in Git is by using the git stash
command. This command allows you to save your uncommitted changes temporarily and revert your working directory to match the HEAD commit. After updating from the remote repository, you can apply your stashed changes back. Here’s how to do it:
git stash
git pull origin main
git stash pop
Output:
On branch main
Your branch is up to date with 'origin/main'.
Already up to date.
First, you run git stash
, which stores your local modifications away and gives you a clean working directory. Next, you pull the latest changes from the remote repository using git pull origin main
. After successfully updating your local branch, you can restore your changes with git stash pop
. This command re-applies your stashed changes to your working directory.
Using git stash
is particularly useful when you’re in the middle of a feature or bug fix and need to quickly sync with the remote repository without committing your changes. Just remember that if there are conflicts when you apply your stash, Git will notify you, and you’ll need to resolve them.
Using Git Checkout
Another method to ignore local changes is by using git checkout
. This command can be particularly useful when you want to discard local changes to specific files or revert them to their last committed state. Here’s how you can do it:
git checkout -- <file>
git pull origin main
Output:
Already up to date.
In this example, replace <file>
with the name of the file you want to discard changes for. The git checkout -- <file>
command will revert the specified file back to its last committed state, effectively ignoring any local changes. After that, you can safely pull updates from the remote repository using git pull origin main
.
This method is beneficial when you have made changes to a file but realize you want the latest version from the repository instead. However, be cautious: once you run this command, all uncommitted changes to that specific file will be lost permanently.
Using Git Reset
If you want to ignore all local changes and revert your entire working directory to the last commit, you can use git reset
. This command is powerful and should be used with caution, as it will discard all local changes. Here’s how to do it:
git reset --hard HEAD
git pull origin main
Output:
Already up to date.
The git reset --hard HEAD
command resets your working directory and index to match the most recent commit. After executing this command, all local changes will be lost. Once your working directory is clean, you can pull the latest changes from the remote repository with git pull origin main
.
This method is particularly useful when you want to start fresh without any local modifications. However, ensure that you have backed up any important changes before executing this command, as it cannot be undone.
Using Git Update-Index
For a more selective approach, you can use git update-index
to ignore changes to specific files. This command allows you to mark files as unchanged in the index, meaning Git will not track changes to them until you decide otherwise. Here’s how to do it:
git update-index --assume-unchanged <file>
git pull origin main
Output:
Already up to date.
By running git update-index --assume-unchanged <file>
, you tell Git to ignore any changes made to the specified file. This is particularly useful for configuration files or other files that may differ in local environments but should remain unchanged in the repository. After marking the file this way, you can pull the latest updates from the remote without worrying about your local changes.
If you later decide to track changes to the file again, you can run:
git update-index --no-assume-unchanged <file>
This command will revert the file back to being tracked by Git.
Conclusion
Ignoring local file changes in Git is essential for maintaining a smooth workflow, especially when collaborating with others. Whether you choose to stash your changes, revert files, reset your working directory, or selectively ignore files, each method has its advantages. By mastering these techniques, you can confidently sync with the remote repository without the fear of losing your local modifications. As you continue to work with Git, these strategies will become invaluable tools in your version control arsenal.
FAQ
-
What is
git stash
used for?
git stash is used to temporarily save uncommitted changes in your working directory, allowing you to pull updates from a remote repository without losing your local modifications. -
Can I ignore changes to multiple files at once?
Yes, you can usegit update-index --assume-unchanged <file1> <file2>
to ignore changes to multiple files simultaneously. -
What happens when I run
git reset --hard HEAD
?
Runninggit reset --hard HEAD
will discard all local changes and reset your working directory to the last committed state. -
How can I see which files are marked as unchanged?
You can use the commandgit ls-files -v
to list files and see which ones are marked as unchanged. -
Is it possible to recover changes after using
git reset --hard
?
No, once you rungit reset --hard
, all uncommitted changes are permanently lost and cannot be recovered.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedInRelated Article - Git Checkout
- Git Checkout VS Pull
- Difference Between Git Checkout and Git Clone
- How to Checkout a Remote Git Branch
- How to Rollback to an Old Commit in a Public Git Repository
- Difference Between Git Checkout --Track Origin/Branch and Git Checkout -B Branch Origin/Branch