How to Understand the Git Conflict Markers
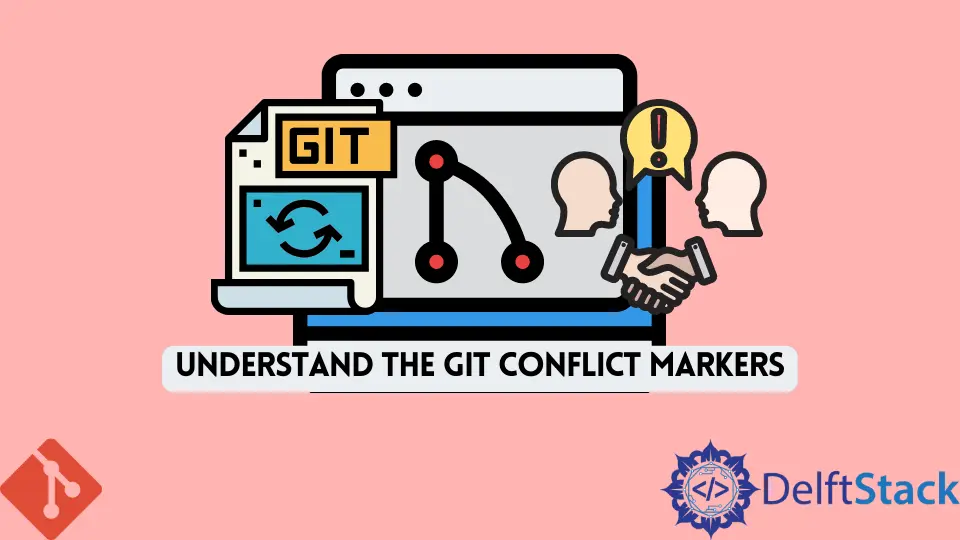
In this article, we will discuss git conflict markers.
Understand the Git Conflict Markers
When pulling changes from the remote repository, you may encounter merge conflicts. The merge conflict file can, at times, be confusing.
A typical merge conflict file looks like this:
<<<<<<< HEAD: file.txt
foo
=======
bar
>>>>>>> cb1abc6bd98cfc84317f8aa95a7662815417802d:file.txt
Let’s discuss the elements in the fence above.
<<<<<<< HEAD:file.txt
foo
=======
The part above shows the file in your local repository. The HEAD
is pointing to our branch or commit.
=======
bar
>>>>>>> cb1abc6bd98cfc84317f8aa95a7662815417802d:file.txt
The part above shows the changes you have introduced from the remote repository. The cb1abc6bd98cfc84317f8aa95a7662815417802d
is the hash
or SHA1sum
of the commit being merged from the remote repository.
This means that when running a git pull
command which is basically a combination of git fetch
and git merge
, the top half shows the local changes. In contrast, the bottom half represents the remote changes being introduced to the local repository from the remote repository.
When running the git rebase origin/master
command, the upper part represents the upstream changes while the lower half shows the local changes being merged.
You will have to edit those parts manually and then commit the results.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn