How to Stage Deleted Files in Git
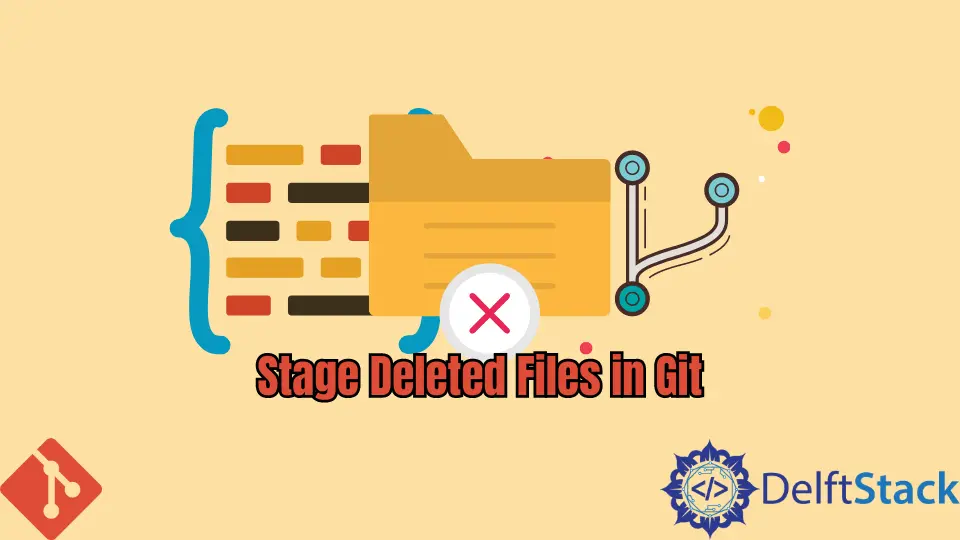
This article discusses the steps necessary to stage a deleted file in Git.
We know that the rm
command can delete a file without removing it from the working directory. So, how do we stage the deleted file for commit?
Stage Deleted Files in Git
For easier context, let’s simulate a situation where we need to stage a file we had deleted. To do that, we will navigate to our repository and delete one of the files.
We will run:
$ rm sample.php
Let’s check our index:
$ git status
Newer Git versions allow us to stage the deleted file with the git add
command. Make sure you have Git 2 and newer.
You can run the command below to check your version:
$ git version
git version 2.35.1.windows.2
We can proceed to add the file, as shown below:
$ git add sample.php
On inspecting our index, the sample.php
file should be staged for commit. Let’s check if this is the case.
There you have it. Our deleted sample.php
file is ready for commit.
In a nutshell, newer versions of Git allow us to stage a deleted file for commit using the git add
command. Git treats it like a modified or added file.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn