How to Create an Environment Variable File in Docker
- Create a Nginx Project
- Define a Nginx Image
- Create an Environment Variable File
- Build an Image
-
Run a Nginx Container Using
docker run
-
Set a
.env
File Usingdocker-compose
-
Run a Nginx Container Using
docker-compose
- Conclusion
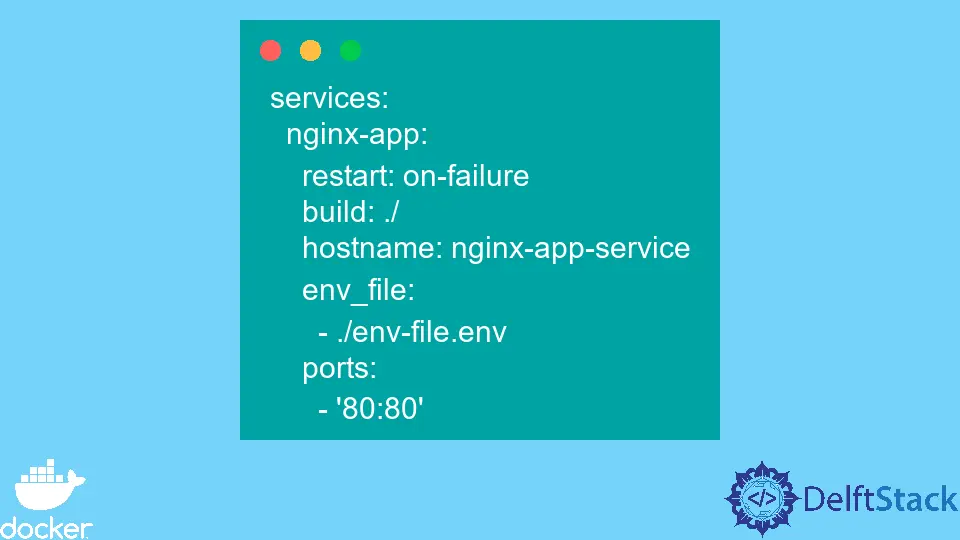
Environment variables are provided to an application in the form of key-value pairs. We can view these variables as metadata that provide information to configure or run an application.
For example, when developing an application that integrates with a communication service such as Twilio or Vonage, you must provide the API keys for the communication service to work.
These API keys can be provided as environment variables, and the application will read the values of the keys from the .env
file.
In this tutorial, we will learn the different ways that we can use to create an environment variable file in docker.
Create a Nginx Project
Open WebStorm IDE and select File>New>Project
. Then, select Empty project
and change the project name to dockerfile-env-file
or enter any preferred name.
Create an index.html
file under the current folder and copy and paste this code into the file.
<!doctype html>
<html lang="en">
<head>
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC"
crossorigin="anonymous">
</head>
<body>
<div class="container-fluid">
<div class="row">
<div class="col-md-3">
</div>
<div class="col-md-6">
<form class="mt-5">
<div class="mb-3">
<label for="exampleInputEmail1" class="form-label">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp">
<div id="emailHelp" class="form-text">We'll never share your email with anyone else.</div>
</div>
<div class="mb-3">
<label for="exampleInputPassword1" class="form-label">Password</label>
<input type="password" class="form-control" id="exampleInputPassword1">
</div>
<div class="mb-3 form-check">
<input type="checkbox" class="form-check-input" id="exampleCheck1">
<label class="form-check-label" for="exampleCheck1">Check me out</label>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
</div>
<div class="col-md-3">
</div>
</div>
</div>
</body>
</html>
In this file, we have used Bootstrap 5 to create a web page that displays a form containing two input fields, a checkbox, and a button. This web page will be displayed when we run a container.
Define a Nginx Image
Create a file named Dockerfile
under the current folder and copy and paste the following instructions into the file.
FROM nginx:1.22.0-alpine
COPY . /usr/share/nginx/html
FROM
- Creates a custom image by following this command’s instructions. Note that this must be the first instruction in theDockerfile
.COPY
- Copies all the files and folders in the host’s current directory to the image file system. When using an Nginx image, the files and directories must be copied to:/usr/share/nginx/html
.
Create an Environment Variable File
Create a file named env-file.list
under the current folder and copy and paste the following key-value pairs into the file.
VERSION=1.0.0
FOO=bar
DESC=This is an Nginx service
DATE=12/11/2022
We will use this file to load the environment variables into a running container. Note that the file’s contents should use the syntax <variable>=value
to set the variable to the assigned value or read the value from the local environment.
When running the container using Dockerfile, we can use any file extension as long as the content adheres to the syntax. As you have noted, this file uses the .list
extension.
Build an Image
To build an Nginx image using the Dockerfile defined above, open a new terminal window on your machine and use the following command to build the image.
~/WebstormProjects/dockerfile-env-file$ docker build --tag env-image:latest .
This command executes the Dockerfile sequentially to build an image with the tag env-image:latest
. The process can be seen from the terminal window, as shown below.
=> CACHED [1/2] FROM docker.io/library/nginx:1.22.0-alpine@sha256:addd3bf05ec3c69ef3e8f0021ce1ca98e0eb21117b97ab8b64127e3ff6e444ec 0.0s
=> [internal] load build context 0.3s
=> => transferring context: 2.45kB 0.0s
=> [2/2] COPY . /usr/share/nginx/html 0.8s
=> exporting to image 1.0s
=> => exporting layers 0.7s
=> => writing image sha256:de1d72539dd9f36eea4a73d47c07d5aa27bb5f693104c00d9d55a52fba4c26a6 0.1s
=> => naming to docker.io/library/env-image:latest
Run a Nginx Container Using docker run
On the same window, use the command below to run a container with the name env-container
.
~/WebstormProjects/dockerfile-env-file$ docker run --name env-container -d -p 80:80 --env-file ./env-file.list env-image:latest
Output:
62fdc85504a2632e5d96aacec4c66c3087a6c1254afadf41bf629b474ceac90c
Note that this command uses the flag --env-file
to load the contents of the env-file.list
in the container.
The value of this flag is the location of the environment variable file. In our case, the file is located in the current folder; thus, we have passed the value ./env-file.list
.
To verify that the environment variables in the file were loaded to the container, use the following command to open an interactive shell for the container.
~/WebstormProjects/dockerfile-env-file$ docker exec -it env-container /bin/sh
Output:
/ #
On the new shell terminal, use the following command to print all the environment variables for this container.
/ # printenv
Output:
HOSTNAME=62fdc85504a2
SHLVL=1
HOME=/root
PKG_RELEASE=1
DATE=12/11/2022
DESC=This is an Nginx service
VERSION=1.0.0
TERM=xterm
NGINX_VERSION=1.22.0
PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin
FOO=bar
NJS_VERSION=0.7.6
PWD=/
Note that the environment variables we added in the env-file.list
have been loaded into the container. The variables include DATE
, DESC
, VERSION
, and FOO
.
Set a .env
File Using docker-compose
In the previous section, we learned the first approach of setting a .env
file in docker using the docker run command. In this section, we will learn how to use the docker-compose
to set a .env
file.
The first thing we must do is to change the extension of the env-file.list
to env-file.env
. Docker expects a file with the .env
extension. Note that this is the default extension for environment variables.
After changing the file extension, create a file named compose.yml
under the current folder and copy and paste the following instructions into the file.
services:
nginx-app:
restart: on-failure
build: ./
hostname: nginx-app-service
env_file:
- ./env-file.env
ports:
- '80:80'
Run a Nginx Container Using docker-compose
Open a new terminal window and use the following command to run an Nginx container using docker-compose
.
~/WebstormProjects/dockerfile-env-file$ docker compose up -d
Output:
[+] Building 12.4s (8/8) FINISHED
=> [internal] load build definition from Dockerfile 0.2s
=> => transferring dockerfile: 31B 0.0s
=> [internal] load .dockerignore 0.2s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/nginx:1.22.0-alpine 9.6s
=> [auth] library/nginx:pull token for registry-1.docker.io 0.0s
=> [internal] load build context 0.2s
=> => transferring context: 476B 0.0s
=> CACHED [1/2] FROM docker.io/library/nginx:1.22.0-alpine@sha256:addd3bf05ec3c69e 0.0s
=> [2/2] COPY . /usr/share/nginx/html 1.1s
=> exporting to image 1.0s
=> => exporting layers 0.7s
=> => writing image sha256:e4bd638d4c0b0e75d3e621a3be6526bfe7ed4543a91e68e4829e5a7 0.1s
=> => naming to docker.io/library/dockerfile-env-file_nginx-app 0.1s
Use 'docker scan' to run Snyk tests against images to find vulnerabilities and learn how to fix them
[+] Running 2/2
⠿ Network dockerfile-env-file_default Created 0.2s
⠿ Container dockerfile-env-file-nginx-app-1 Started 2.9s
Note that since we have specified the env_file
in the docker compose.yaml
, we do not need to specify the command when running the container.
This command will use the docker-compose
file to run a container and automatically use the env-file.env
file in the current directory to load the variables in the container.
To verify that the variables in the .env
file were loaded into the container, use the following command to execute an interactive shell from the container.
~/WebstormProjects/dockerfile-env-file$ docker exec -it dockerfile-env-file-nginx-app-1 /bin/sh
Output:
/ #
On the new shell terminal, use the following command to print all the environment variables in the new container.
/ # printenv
Output:
HOSTNAME=nginx-app-service
SHLVL=1
HOME=/root
PKG_RELEASE=1
DATE=12/11/2022
DESC=This is an Nginx service
VERSION=1.0.0
TERM=xterm
NGINX_VERSION=1.22.0
PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin
FOO=bar
NJS_VERSION=0.7.6
PWD=/
We can see from the output that all the variables we added in the .env
file were successfully loaded into the container. The variables include DATE
, DESC
, VERSION
, and FOO
.
Conclusion
In this tutorial, we’ve learned how to set environment variables using an environment variable file. In the first approach, we used the flag --env-file
with the docker run command to load the variables into a container. In the second approach, we added the env_file
instruction in the compose.yaml
file automatically load the files in a container.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub