How to Rename an Image in Docker
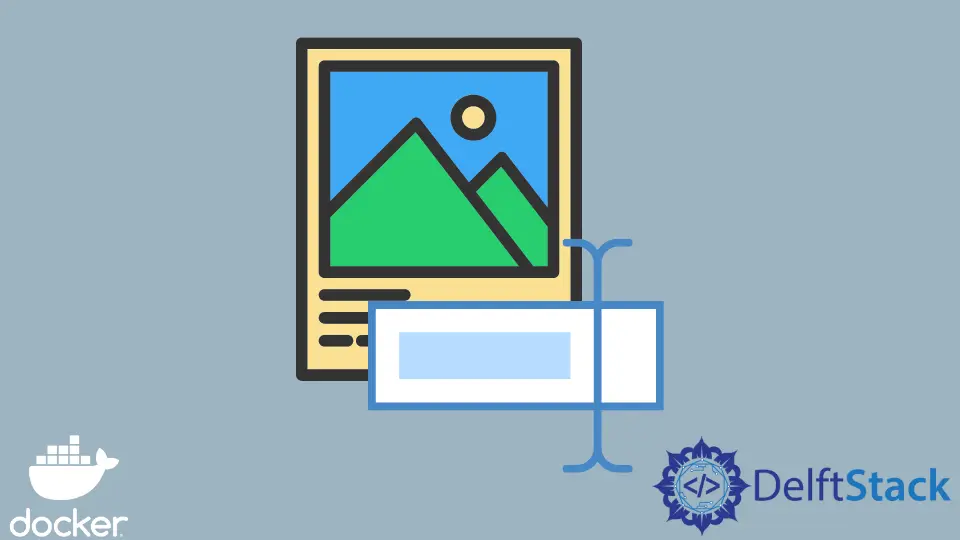
In Docker, we can use a simple way to rename an image without rebuilding it from scratch. This article will discuss how to rename an image using Docker.
Rename an Image in Docker
We can rename an image or change a repository name by changing the image tags. We recall that tags are just human-readable aliases for the full image name.
docker image tag server:latest sample/server:latest
or
docker image tag d583c3ac35fd sample/server:latest
As a shorthand approach, we can run the following snippet:
docker tag d58 sample/server:latest
The argument d58
represents the first three characters of the IMAGE ID. In this case, those few characters are all we need.
Remove Old Images as Best Practice
Finally, we can remove the old image to have as many tags associated with the same image as you like. If we don’t like the old image name, we can remove it after we have retagged it:
docker rmi server
The command will remove the alias/tag
tagging format. However, since d58...
has other names, Docker won’t delete the actual image.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn