How to Create Directory With Mkdir Command in a Container With Dockerfile
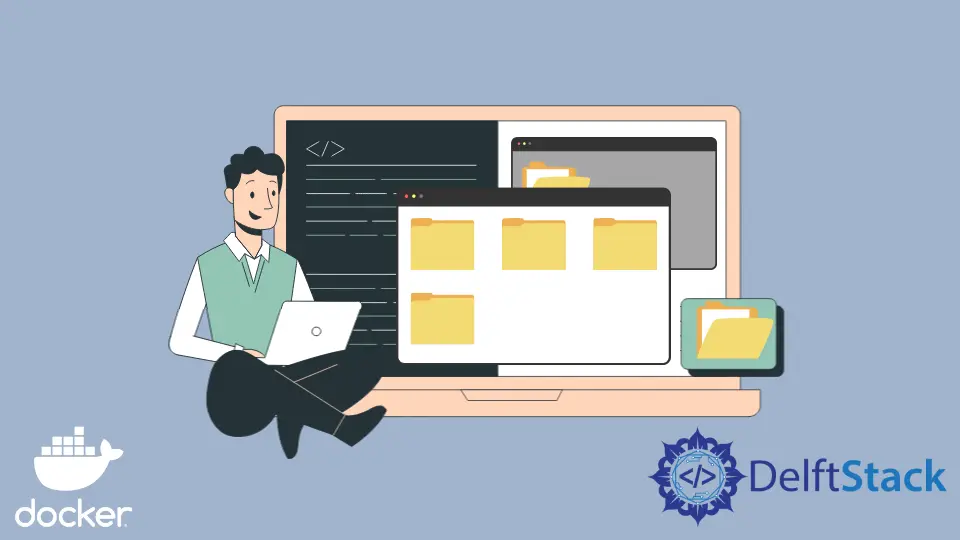
Docker containers have become the de facto way to manage software and dependencies in different environments. When working with real applications, there is no doubt that you’ll need to create a Dockerfile before you can build your applications container image.
Create Directory With mkdir
Command in a Container With Dockerfile
Apart from merely allowing developers to assemble commands necessary to create Docker images, there is so much more that we can do with Dockerfiles. To successfully create a Docker image using a Dockerfile, you must know some basic commands.
Some of the most used commands include:
FROM
- Creates a layer of the parent image/base image that will be used.WORKDIR
- allows us to set the working directory.COPY
- allows us to copy current directory contents into a directory in the container.PULL
- Adds files from your Docker repository.RUN
- Command to be executed when we want to build the image.CMD
- specifies what command to run when the container starts.ENV
- Defines environmental variables used during the build.ENTRYPOINT
- specifies what command to run when the container starts.MAINTAINER
- Specifies the author of the image.
Using the commands above, we can create a Dockerfile such as the one below that uses Python as the base image.
Code:
# base image
FROM Python
# Set your working directory
WORKDIR /var/www/
# Copy the necessary files
COPY ./app.py /var/www/app.py
COPY ./requirements.txt /var/www/requirements.txt
# Install the necessary packages
RUN pip install -r /var/www/requirements.txt
# Run the app
CMD ["echo", "Hello, Developer"]
This Dockerfile is part of a simple Flask application that only prints a message on the console. Here is the requirements.txt
file if you’d be interested in creating the same application.
click==8.0.4
Flask==2.0.3
gunicorn==20.1.0
itsdangerous==2.1.0
Jinja2==3.0.3
MarkupSafe==2.1.0
Werkzeug==2.0.3
The main file app.py
containing that main application file is shown below.
Code:
from flask import Flask
app = Flask(__name__)
def hello():
print("Hello, this is a simple Flask application")
hello()
Now, to create a directory with the mkdir
command inside the file system of our Docker container, we will use the RUN
command as shown below.
# base image
FROM Python
# Set your working directory
WORKDIR /var/www/
# Copy the necessary files
COPY ./app.py /var/www/app.py
COPY ./requirements.txt /var/www/requirements.txt
# Install the necessary packages
RUN pip install -r /var/www/requirements.txt
RUN mkdir -p /var/www/new_directory
# Run the app
CMD ["echo", "Hello, Developer"]
The command RUN mkdir -p /var/www/new_directory
allows you to create a directory named new_directory
inside the Docker file system that we will eventually build using an image built using the above Docker file.
However, we will begin by building a Docker image based on Python as the base image by running the command below.
isaac@DESKTOP-HV44HT6:~/my-app$ docker build -t new_image .
Output:
Confirm we have successfully built a Docker image from the Docker file using the docker images
command below.
$ docker images
Output:
REPOSITORY TAG IMAGE ID CREATED SIZE
new_image latest 7ab964c50876 13 minutes ago 932MB
Now that we have a Docker image in place, we can go ahead and create a Docker container and confirm if the directory named new_directory
was created. As shown below, we will need to launch Bash
within the container to navigate directories within the Docker container.
$ docker run -it new_image bash
root@ea42f35d5404:/var/www#
You’ll notice that the new_directory
was created after listing the files in this directory. In addition to this, we can also navigate the directory itself and even create new files within it.
root@ea42f35d5404:/var/www# ls
app.py new_directory requirements.txt
root@ea42f35d5404:/var/www# cd new_directory
root@ea42f35d5404:/var/www/new_directory# ls
root@ea42f35d5404:/var/www/new_directory# touch new_file
root@ea42f35d5404:/var/www/new_directory# ls
new_file
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn