How to Update Existing Images With Docker Compose
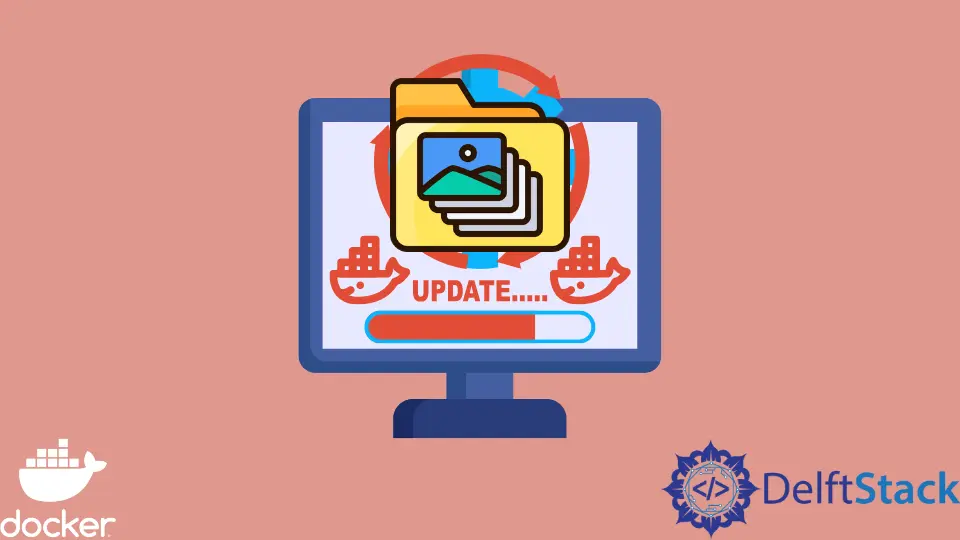
Docker containers are designed to be disposable and easily replaced. Therefore, we should pull the updated image and launch a fresh instance of the container whenever the base image for a container receives an update.
This article will discuss how to update existing images with docker-compose
.
Update Existing Images With Docker Compose
Ensure all photos have been downloaded before updating the containers with the new images to minimize the time spent in an intermediate state or being in the middle in the event that an image download fails.
-
Update the latest image using the following snippet.
docker-compose pull
-
Restart the containers using the command below. The snippet above will only recreate changed containers.
We can also append the
--force-recreate
on this command; however, this will only recreate containers that are not changed.docker-compose up -d --remove-orphans
-
As an optional step, remove any obsolete images.
docker image prune
For the last step, we don’t need the use of the
-f
or--force-recreate
parameters, as this could lead to destructive side effects on our images.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn