Timezone in Django
Vaibhav Vaibhav
Jun 14, 2021
Django
Django DateTime
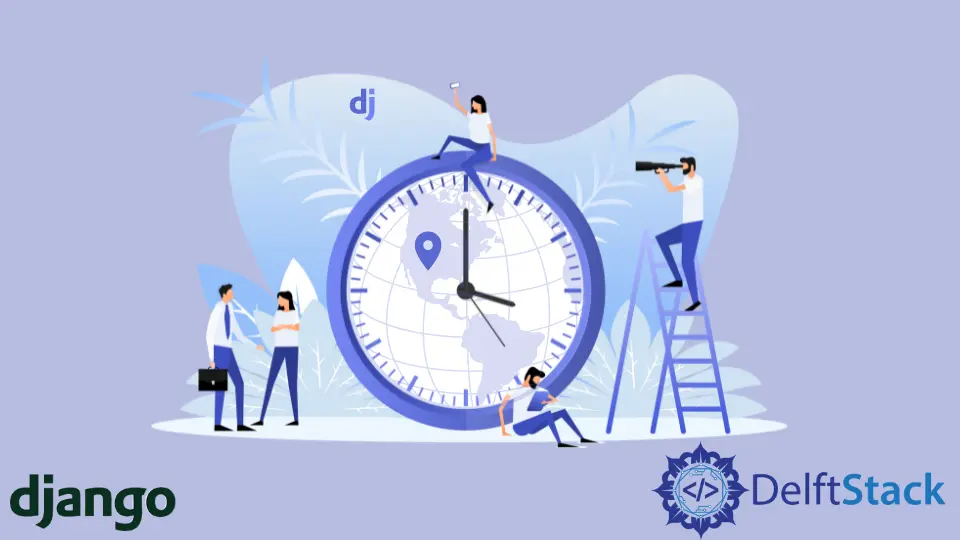
When building software, apart from creating a secure and robust back end, and an intuitive front end design, we have to focus on date and time. Date and time might not be essential elements in most software, but they still play a vital role in enhancing user experience.
In Django, setting up a local time zone is relatively simple. By default, Django uses UTC
or Coordinated Universal Time
or Universal Time Coordinated
.
In settings.py
, we have to configure the TIME_ZONE
variable to set the local time zone.
TIME_ZONE = "Asia/Kolkata"
TIME_ZONE = "Asia/Beirut"
TIME_ZONE = "Europe/London"
TIME_ZONE = "Europe/Istanbul"
The value follows the following format - Area/Location
. You can find all the valid values here.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Vaibhav Vaibhav