How to Use the Include Tag in Django
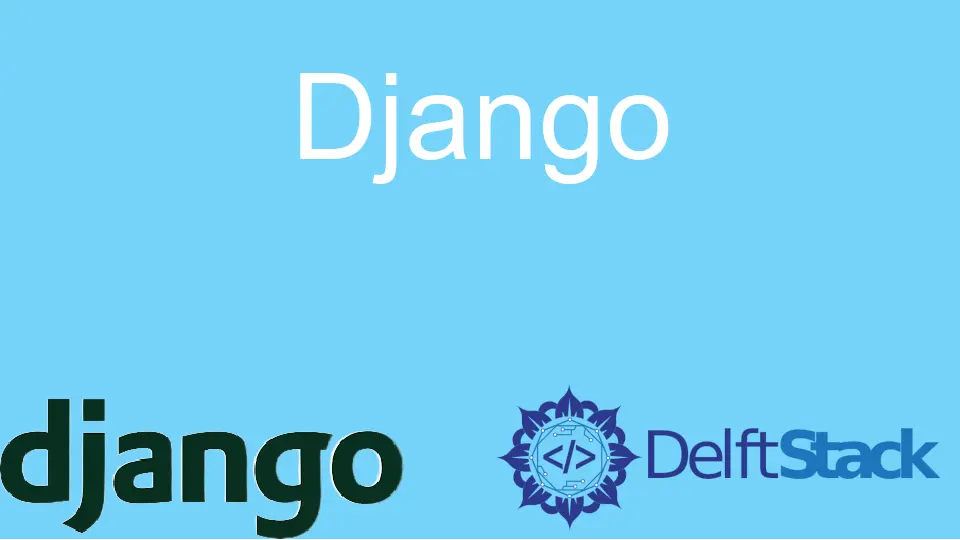
This tutorial aims to quickly and easily teach how to use the include
tag inside the individual HTML file in Django.
Import External File Inside the HTML File Using the include
Tag in Django
In the Django documentation, there are different built-in template tags that we can use. And the extends
tag is part of the template inheritance.
The include
tag is also part of the template inheritance. The include
tag brings a smarter way to avoid redundant code and helps add an external file to a little piece of code.
For example, if we have a home page with a navbar and integrate multiple pages around a whole website, we will need to add it on different pages. However, it is a time-consuming process to add a navbar on every page separately.
In that case, we are not going to add a navbar individually. We will need to create our base.html
and navbar.html
files, write a navbar script in these files, then we can use it where we want to replace it using the include
tag.
According to the documentation, the include
tag loads and renders the template with the current context. This is a way to include other templates within a template.
Now, we will create a new HTML file using the navbar.html
file employing the include
tag. We have to use this tag in the HTML body
tag, and we will need to use jinja templating inside the HTML code.
Jinja templating helps us import variables and apply inheritance inside the HTML file. We can see the include
tag needs opening and closing curly brackets, and around them, we will write the include
tag, and after one space, write the file name that we need to replace.
If we run our server, we can now see that we have a navbar on another page.
You have learned how easily you can use the include
tag and include an external HTML file in your Django project. You can get more information from here.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn