How to Represent One to Many Relationship in Django
- Represent One to Many Relationship using a Junction / Intermediatory Model
- Represent One to Many Relationships using Foreign Keys
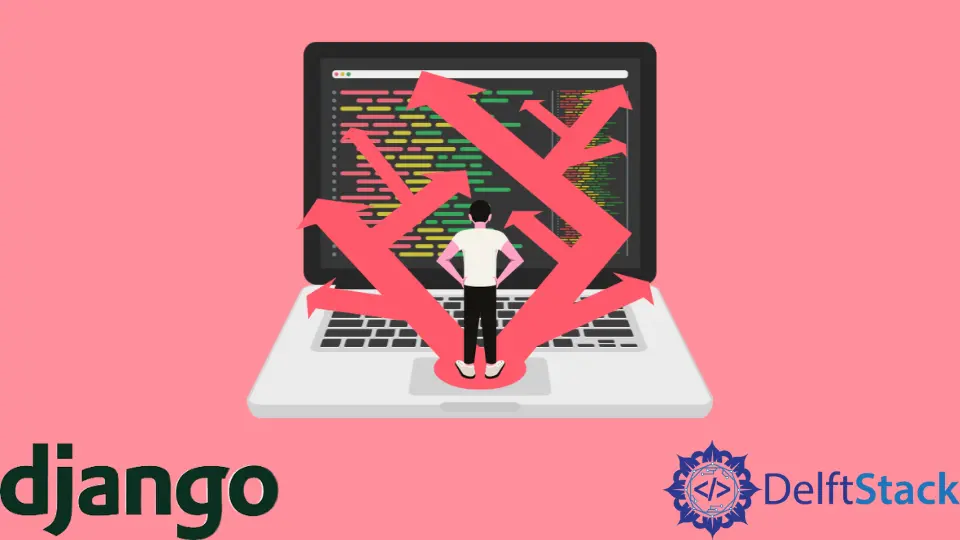
Databases play a key role almost everywhere, and it’s no surprise that web development is one such place. Building tables and setting up relationships between tables using SQL is a simple task, but Django makes it even simpler.
This article will introduce how to represent one to many relationships in Django.
Represent One to Many Relationship using a Junction / Intermediatory Model
Refer to the following models’ definitions.
class Number(models.Model):
number = models.CharField(max_length=10)
class Person(models.Model):
name = models.CharField(max_length=200)
class PersonNumber(models.Model):
person = models.ForeignKey(Person, on_delete=models.CASCADE, related_name="numbers")
number = models.ForeignKey(Number, on_delete=models.CASCADE, related_name="person")
The Number
model has a field number
to store a phone number.
The Person
model has a field name
for the person’s name.
PersonNumber
is a junction or intermediatory model for Number
and Person
. The foreign keys have a cascading relationship with the referred objects. This model can be used to set up a one-to-many relationship as well as a many-to-many relationship.
The default primary key for all the models is id
, an integer auto-field.
Since a phone number is only associated with a single person, but a person can have more than one phone number, this is a one-to-many relationship. PersonNumber
will be used to represent this relationship.
If a variable person
is storing a Person
object, we can easily access all the phone numbers of this person using the following statement.
numbers = person.numbers.objects.all()
This statement will return a QuerySet
of Number
objects.
Consider this example.
ID | Person | Number |
---|---|---|
1 | 1 | 1 |
2 | 1 | 2 |
3 | 1 | 3 |
4 | 1 | 4 |
5 | 2 | 5 |
6 | 2 | 6 |
7 | 3 | 7 |
A person with id
1
has four phone numbers with id
1
, 2
, 3
, and 4
.
A person with id
2
has two phone numbers with id
5
, and 6
.
A person with id
3
has only one phone number with id
7
.
Represent One to Many Relationships using Foreign Keys
Refer to the following models’ definitions.
class Person(models.Model):
name = models.CharField(max_length=200)
class Number(models.Model):
person = models.ForeignKey(Person, on_delete=models.CASCADE)
number = models.CharField(max_length=10)
The Person
model has a field name
for the person’s name.
The Number
model has a field number
to store a phone number and a foreign key reference to the Person
model. This field would store the owner of this number. The foreign key has a cascading relationship with the referenced model, Person
.
Using this structure, we can easily associate each Number
object with its respective owner using the foreign key reference.
If a variable person
is storing a Person
object, we can access all the numbers associated with this person using the following statement.
numbers = Number.objects.filter(person=person)
This statement will return a QuerySet
of Number
objects.