How to Rollback the Last Database Migration in Django
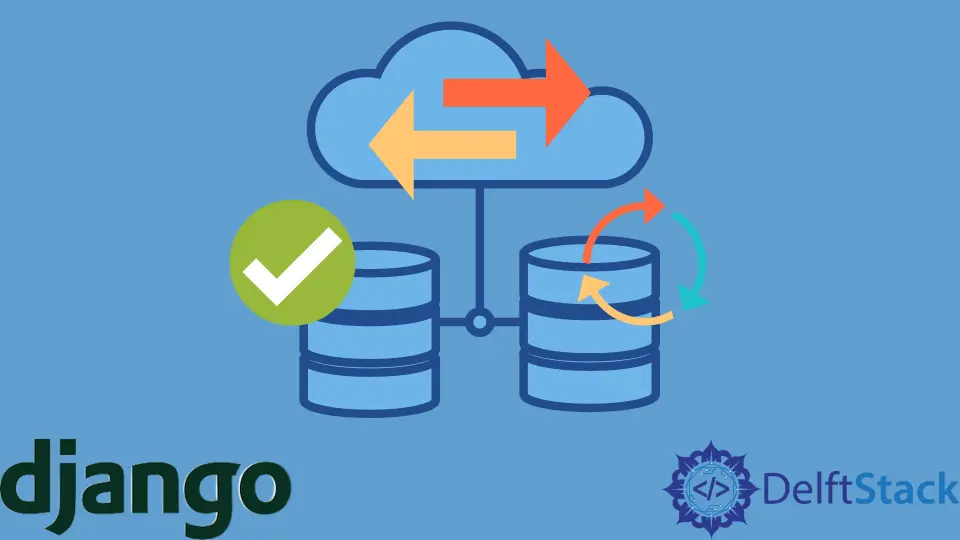
When working with Django Models, we always have to use the migration function and migrate it if we change the models. There are certain situations when we have to revert or reverse a migration. Django makes it easy to revert a migration. This article will show you the way to rollback last database migration in Django projects.
Rollback Django Migration Using migrate
Command
To revert a migration, we can use the migrate
command that the Django’s manage.py
file provides us. Consider a Django App System
and two migration files inside this application’s migrations
folder. Let the two migration files be 0005_second_last_migration
and 0006_last_migration
; note that 0006 is the latest migration that has been applied.
If we have to revert back to 0005 migration from 0006 migration, we will run the following command:
python manage.py migrate System 0005
--- OR ---
python manage.py migrate System 0005_second_last_migration
It’s necessary to mention the application name, and we can use both the migration number and the file name to revert a migration.
If we have to reverse all the migrations of this Django application System
, we will use the command below:
python manage.py migrate System zero
Remember, a migration can be irreversible sometimes. Generally, this condition arises when some significant changes have been made to the Django Models. When we try to revert back to such a migration, Django will raise an IrreversibleError
.