How to Reset Database in Django
- Reset the SQLite3 Database in Django
- Reset the Whole Database in Django
- Reset an App Database Tables in Django
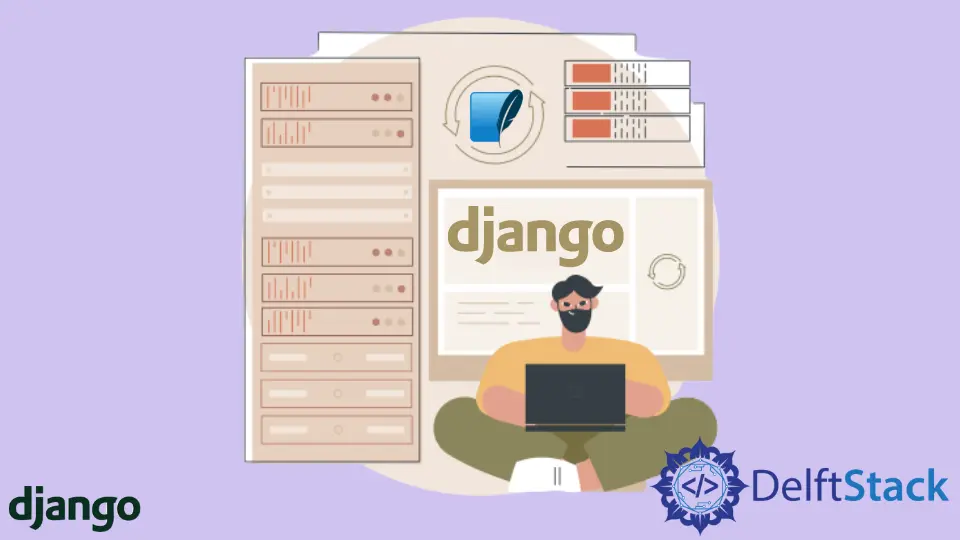
When working with databases, we often end up in situations where we have to reset the whole database. The possible reasons could be the addition or removal of some database tables, changes in the database design, issues on logic and relationships, or the database got populated with too much useless data. Whatever the case may be, Django makes it really easy to deal with this problem.
Moreover, Django provides us with few commands that can handle this for us. One of these commands can reset database in Django, and we’re here to demonstrate how you can use it.
Reset the SQLite3 Database in Django
If you’re using the SQLite3 Database for your Django project and you have to reset it, follow the steps below:
-
Delete the
db.sqlite3
file. If this file contains important data, you might want to settle a backup for those. -
Delete all the
migrations
folder inside all the Django applications. -
Make migrations for all the Django applications using the
python manage.py makemigrations
command. There might be instances where migrations are not made for the applications; in this case, add the application names to this command like thispython manage.py makemigrations MyAppOne MyAppTwo MyAppThree
. -
Lastly, migrate the migrations using this command:
python manage.py migrate
.
Reset the Whole Database in Django
If we have to reset the whole database completely, we will use the following command: (Note: After using this code, all the existing superusers will be deleted as well.)
python manage.py flush
Reset an App Database Tables in Django
If we have to remove the database tables of a Django application, we will use the command below. The following code reverses all the migrations for that particular application:
python manage.py migrate MyApp zero