How to Reset Admin Password in Django
- Understanding the Django Admin Panel
- Method 1: Using the Django Shell to Reset the Admin Password
- Method 2: Changing the Admin Username
- Method 3: Using Django’s Built-in Commands
- Conclusion
- FAQ
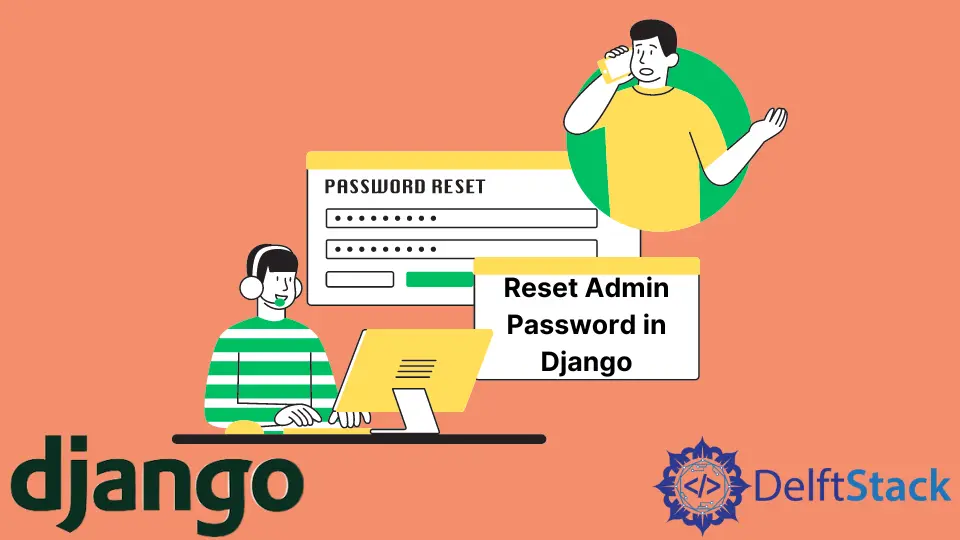
When working with Django, the admin panel is a powerful interface that allows you to manage your application’s data. However, there may come a time when you forget your admin password or need to change it for security reasons.
In this article, we will walk you through the steps to reset the admin password in Django and even modify the admin username if needed. Whether you’re a seasoned developer or just starting out, these methods will help you regain access to your admin panel quickly and efficiently.
Understanding the Django Admin Panel
The Django admin panel is an automatically-generated interface that provides a user-friendly way to manage your application’s data. It allows you to perform CRUD (Create, Read, Update, Delete) operations on your models with ease. By default, the admin panel is accessible to users with superuser permissions, which means they have full control over the application. This is why it’s crucial to maintain the security of your admin account, including the password.
Method 1: Using the Django Shell to Reset the Admin Password
One of the most straightforward ways to reset your admin password is through the Django shell. This method requires access to your project’s environment and allows you to directly modify the user’s password. Follow these steps:
- Open your terminal or command prompt.
- Navigate to your Django project directory.
- Activate your virtual environment if you are using one.
- Run the Django shell with the command:
python manage.py shell
- Once in the shell, execute the following code to reset the password:
from django.contrib.auth.models import User
user = User.objects.get(username='admin')
user.set_password('new_password')
user.save()
In this code snippet, we first import the User model from Django’s authentication system. Then, we retrieve the admin user by their username. The set_password
method is used to change the password securely, followed by saving the user instance to apply the changes. This method ensures that the password is hashed properly, maintaining your application’s security.
Method 2: Changing the Admin Username
Sometimes, you may want to change the admin username for various reasons, such as security concerns or simply to make it more memorable. You can also achieve this through the Django shell. Here’s how:
- Open your terminal and navigate to your Django project directory.
- Activate your virtual environment if applicable.
- Launch the Django shell:
python manage.py shell
- Execute the following code to change the admin username:
from django.contrib.auth.models import User
user = User.objects.get(username='admin')
user.username = 'new_admin_username'
user.save()
In this example, we again import the User model and retrieve the admin user. We then change the username
attribute to the new desired username and save the changes. This method is beneficial for maintaining security, especially if the original username is widely known.
Method 3: Using Django’s Built-in Commands
Django also provides built-in management commands that can be used to reset the admin password. This method is particularly useful if you prefer not to interact with the shell directly. Here’s how to do it:
- Open your terminal and navigate to your Django project directory.
- Activate your virtual environment if necessary.
- Run the following command to reset the password:
python manage.py changepassword admin
Output:
Changing password for user 'admin'.
New password:
Password changed successfully.
When you run this command, Django prompts you to enter a new password for the specified user (in this case, ‘admin’). This method is straightforward and ensures that the password is changed securely without needing to write any additional code.
Conclusion
Resetting the admin password and changing the username in Django is a straightforward process that can be accomplished using various methods. Whether you choose to use the Django shell or built-in commands, each method provides a secure way to manage your admin account. Keeping your admin credentials secure is vital for protecting your application, so be sure to follow these steps whenever you need to update your credentials. With this knowledge, you can confidently manage your Django application’s admin panel.
FAQ
-
how do I access the Django admin panel?
You can access the Django admin panel by navigating tohttp://yourdomain.com/admin
in your web browser and logging in with your admin credentials. -
can I reset the admin password without the Django shell?
Yes, you can reset the admin password using Django’s built-in management commands directly from the terminal. -
what should I do if I forget my admin username?
If you forget your admin username, you can retrieve it by querying the User model in the Django shell or checking the database directly. -
is it safe to change the admin password frequently?
Yes, changing the admin password regularly is a good security practice to help protect your application from unauthorized access. -
can I customize the Django admin panel?
Yes, Django provides various customization options for the admin panel, including changing the layout, adding custom actions, and modifying the appearance.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn