How to Completely Uninstall a Django App
- Step 1 - Move the Required Code and Files to a Safe Location
- Step 2 - Fix All the Imports and File Paths
-
Step 3 - Empty
models.py
and Make Migrations -
Step 4 - Fix the
settings.py
File - Step 5 - Delete the App Folder
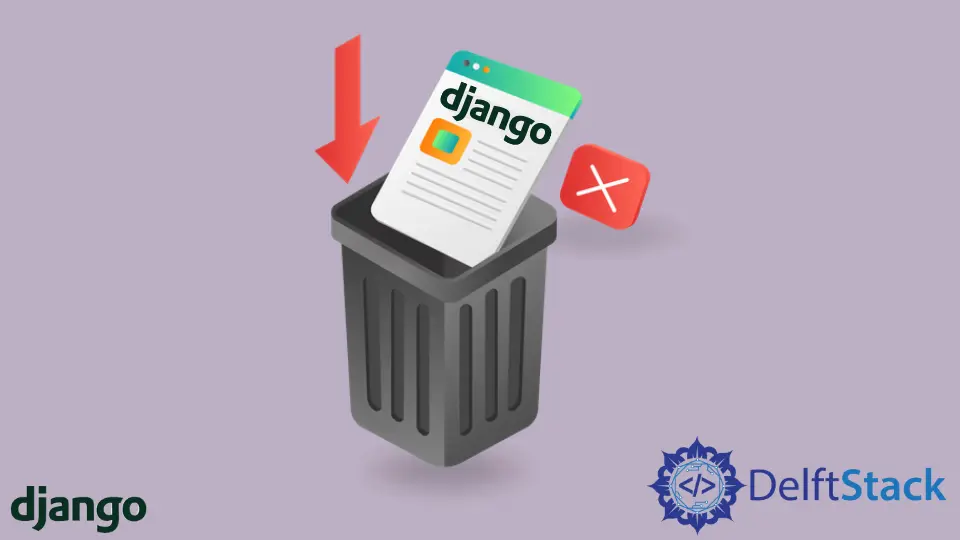
Often, we have to remove a Django app from a specific project entirely when working on big Django projects - this happens when changes occur in a business plan, project design, or the architecture itself.
The simplest yet most dangerous way to delete a Django App is to delete the app folder directly. The Reason? The models inside that application might be linked to the ones from other applications. That application might have some classes, views, functions, or static files on which other software are dependent.
In this article, you’ll learn how to completely uninstall a Django App without running into issues afterward.
Step 1 - Move the Required Code and Files to a Safe Location
One of the most significant steps is to safely move the code and files on which some of the features of your application are dependent or some other Django Apps themselves.
Step 2 - Fix All the Imports and File Paths
After moving the required code and files, fix all the configuration imports like classes, functions, views, and variables, and the file paths to these files. Moreover, there might be some URLs and Models on which other Apps are dependent. So don’t forget to fix those URLs and foreign keys, one-to-one fields, many-to-many fields, and further configurations in the Django Apps.
Step 3 - Empty models.py
and Make Migrations
After making the required changes, empty the models.py
of the App that has to be deleted. Then, make migrations and apply them using the following commands; they will create the new tables and delete all the old tables:
python manage.py makemigrations
python manage.py migrate
Step 4 - Fix the settings.py
File
Remove the application name or the application’s config name from the INSTALLED_APPS
list in settings.py
.
Step 5 - Delete the App Folder
Lastly, after making sure that the app folder only contains the unnecessary files, delete the App folder.