How to Connect Django to MySQL Database
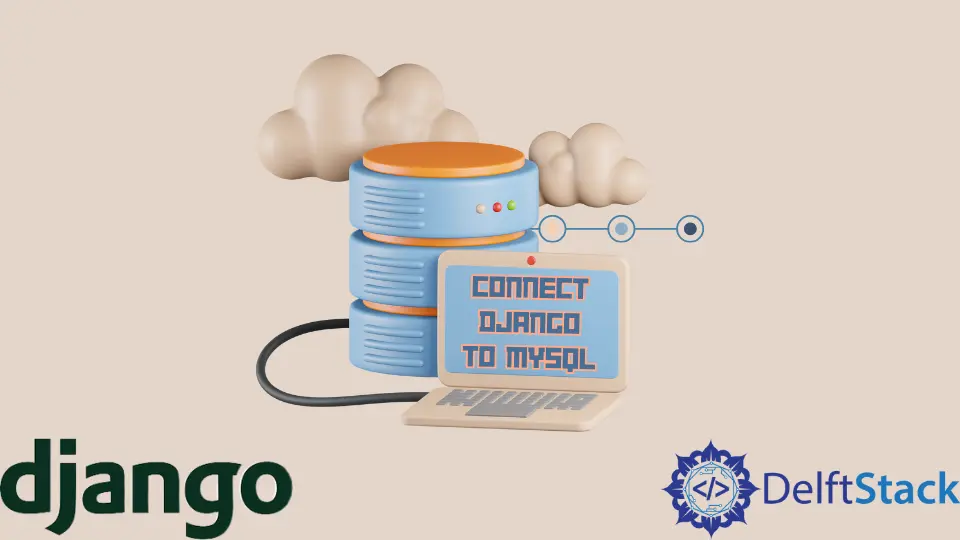
When working with databases, we have a lot of options on the list. We can choose among relational databases or SQL databases such as MySQL, PostgreSQL, SQL Server, SQLite, MariaDB, and non-relational databases or non-SQL databases such as MongoDB and Redis Couchbase.
Since Django is a full-fledged robust web framework, it is compatible with almost all databases. We might have to do some extra work on our end or maybe use some plugins or applications for specific databases, but Django official supports PostgreSQL, MariaDB, MySQL, Oracle, and SQLite.
This article talks about how to connect MySQL to Django.
Initial Setup of Connecting MySQL to Django
Before proceeding with the connection settings, make sure that you have MySQL set up on your system. Make sure that you have an account created and databases created that you wish to connect.
Moreover, you will also require a MySQL Client to interact with the databases using Python (3.X versions).
The MySQL client can be downloaded using the following pip
command.
pip install mysqlclient
Or,
pip3 install mysqlclient
Django MySQL Connection Settings
Django, by default, uses the SQLite database. The connection settings for the same looks like this.
DATABASES = {
"default": {
"ENGINE": "django.db.backends.sqlite3",
"NAME": BASE_DIR / "db.sqlite3",
}
}
To connect Django to the MySQL database, we have to use the following settings.
DATABASES = {
"default": {
"ENGINE": "django.db.backends.mysql",
"NAME": "databaseName",
"USER": "databaseUser",
"PASSWORD": "databasePassword",
"HOST": "localhost",
"PORT": "portNumber",
}
}
The ENGINE
key for MySQL database varies. Apart from that, there are a few extra keys such as USER
, PASSWORD
, HOST
, and PORT
.
NAME |
This key stores the name of your MySQL database. |
USER |
This key stores the username of your MySQL account using which the MySQL database will be connected. |
PASSWORD |
This key stores the password of that MySQL account. |
HOST |
This key stores the IP Address on which your MySQL database is hosted. |
PORT |
This key stores the port number on which your MySQL database is hosted. |
Lastly, make the required migrations using python manage.py makemigrations
and python manage.py migrate
to complete the setup.