How to Change the Label Text Based on the Field Type in Django Forms
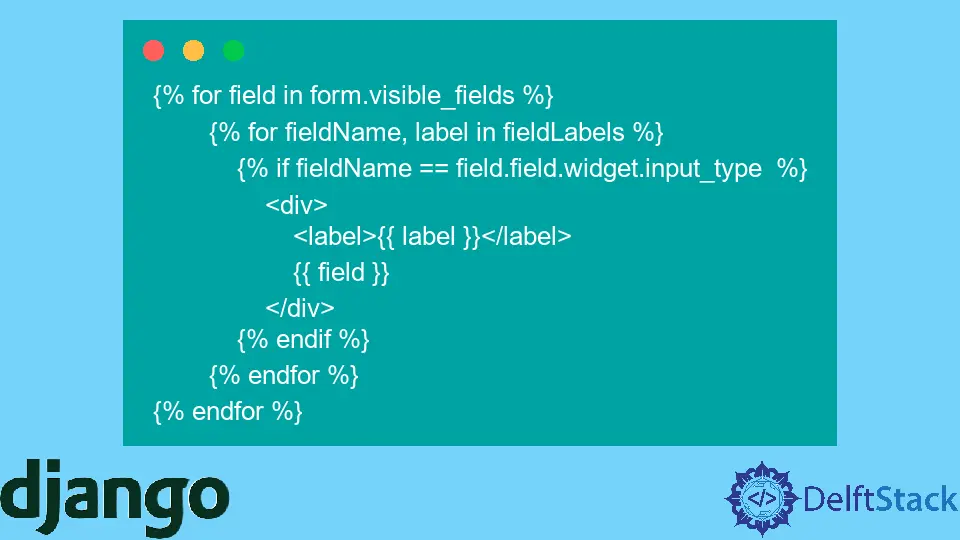
Django is a diverse web framework that comes packed with robust features. It easily handles complex tasks such as authentication, sessions, emailing, etc. It enables us to create dynamic HTML pages, efficiently deal with the databases, design forms quickly, display data in the HTML template using Django’s templating language, etc.
In general, sometimes we have to use some specific value for some other specific value. Think of it as a key-value pair. A particular key has a particular value associated with it.
What if we have to vary the label name based on the field type or display a specific text for a particular field in Django? This article talks about the same.
Change Label Name Using Django Templating Language
In an HTML file, using Django’s templating language, we can access the field type using the following statement.
{{ fieldName.field.widget.input_type }}
Using this trick, we can vary the label names based on the field types.
In the context dictionary of a view of a template, add the following.
"fieldLabels": [
("text", "Label for Text Field"),
("password", "Label for Password Field"),
("file", "Label for File Field"),
("number", "Label for Number Field"),
...,
]
The list above stores tuples in the format ("field type", "label text")
. We can iterate over these tuples in the templates and display the required information based on an if
condition.
Inside a template, do something like this.
{% for field in form.visible_fields %}
{% for fieldName, label in fieldLabels %}
{% if fieldName == field.field.widget.input_type %}
<div>
<label>{{ label }}</label>
{{ field }}
</div>
{% endif %}
{% endfor %}
{% endfor %}
The above code iterates over all the fieldLables
’s values for every form’s field, and if a field name matches, then its respective label text is displayed.