How to Delete a Record of a Model in Django
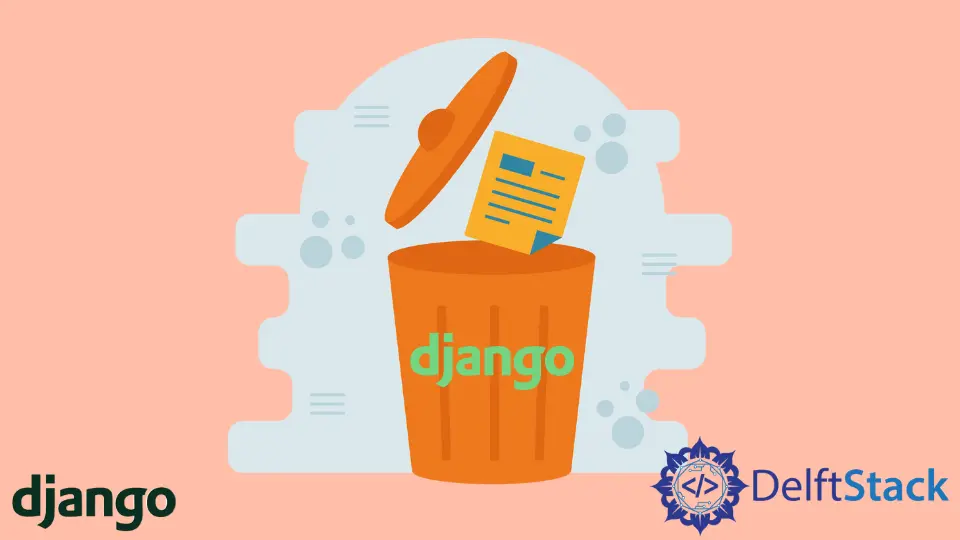
Django is a versatile framework that enables us to build full-stack applications very quickly. Django handles most of the complex and core tasks associated with all the web applications efficiently so that developers don’t have to write them from scratch.
Fortunately, Django handles databases like a charm and makes it really simple to interact with them. Though raw SQL queries can be written in Django, it lets us interact with the database and tables using Python - we can efficiently perform CRUD operations over databases using Python very quickly.
In this article, we’ll introduce the function of the CRUD Operation delete and demonstrate how you can delete a record of a model in Django.
Delete a Record of a Model Using the delete()
Method in Django
All the model objects or instances in Django have a delete()
method, which can be used to delete that record. Now, this delete()
method can be also be used to delete a single record and a bunch of them.
To delete a single record, we’ll use the following code:
record = ModelName.objects.get(id=25)
record.delete()
The get()
method fetches the record with the id
as 25 and then deletes it. But if the record is not found, it raises an exception. To avoid that, we can use a try...except
block as follows:
try:
record = ModelName.objects.get(id=25)
record.delete()
print("Record deleted successfully!")
except:
print("Record doesn't exists")
If we have to delete all the records, we can call this delete()
function on a QuerySet
containing all the records. The code below performs the same operation:
records = ModelName.objects.all()
records.delete()
As mentioned above, we can call this delete()
function on a QuerySet
; this means that we can also call this function on a QuerySet
of some filtered records. Refer to the following code for the same output:
records = ModelName.objects.filter(firstName="Vaibhav")
records.delete()