How to Check the Logged in User in Django
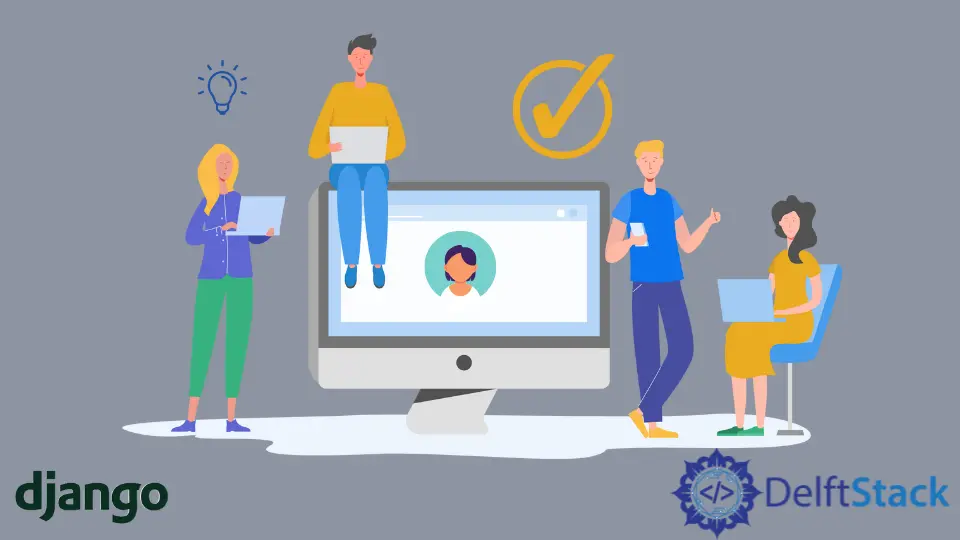
Django comes pre-built with a robust Authentication System. Hence, checking the current logged-in user in Django is pretty straightforward. But it depends where you wish to check for the logged-in user, that is, in views or templates.
In this article, we will discuss how to check the logged-in user for both locations.
Check the Logged in User in Views in Django
In views, we can use the request
to check the logged-in user. A request
has a bunch of information such as the client machine, client IP, request type and data, etc., and one such information is about the user who is making this request.
Refer to the following code
if request.user.is_authenticated:
print("User is logged in :)")
print(f"Username --> {request.user.username}")
else:
print("User is not logged in :(")
We can use request.user.is_authenticated
to check if the user is logged in or not. If the user is logged in, it will return True
. Otherwise, it will return False
.
Check the Logged in User in Templates in Django
Like in views, we can also use the request
inside the templates to check for the logged-in user. The syntax is exactly the same. In templates, we will use Django’s template tags to create an if-else statement
.
<body>
{% if request.user.is_authenticated %}
<p>User is logged in :)</p>
<p>Username --> {{ request.user.username }}</p>
{% else %}
<p>User is not logged in :(</p>
{% endif %}
</body>