Django ArrayField
-
Use
ArrayField
to Add a One-Dimensional Array in Django Models -
Use
ArrayField
to Create a Multi-Dimensional Array in Django Models
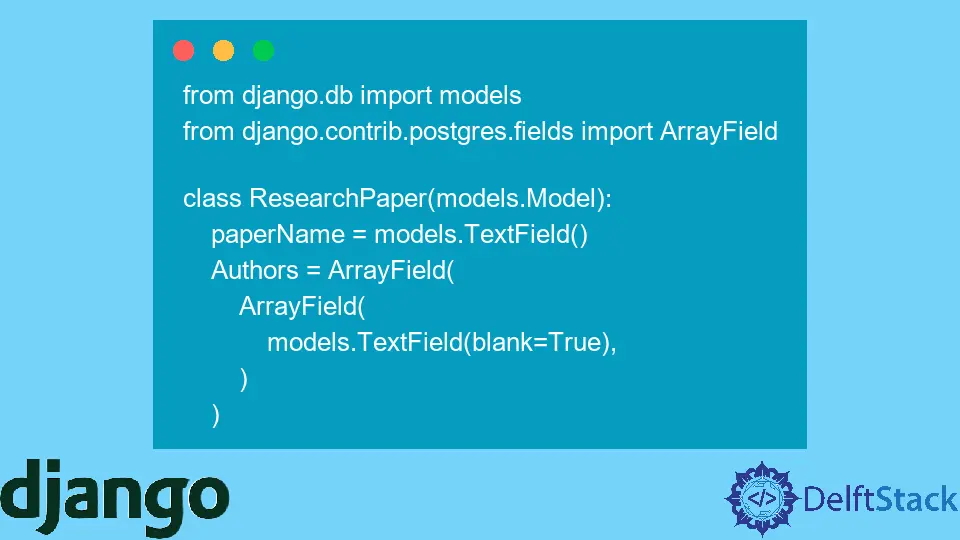
The ArrayField
in Django is similar to an array data structure in other programming languages such as Java, C, C++, etc. It stores multiple values of the same data type.
Before we move ahead with the Arrayfield
in Django, the user should confirm that they are using the PostgreSQL
as a database because the SQLite
database does not support Arrayfield
.
To set up the PostgreSQL
database, users should run the server of PostgreSQL
locally. After that, to connect it with Django, in the settings.py
file of the application, users need to change the DATABASES
array, as shown below.
DATABASES = {
"default": {
"ENGINE": "django.db.backends.postgresql",
"NAME": "<DATABASES_name>",
"USER": "<USER_name>",
"PASSWORD": "<PASSWORD_of_user>",
"HOST": "localhost",
"PORT": "5432",
}
}
In the below image, users can see how we have set up the PostgreSQL
in the settings.py
file.
Use ArrayField
to Add a One-Dimensional Array in Django Models
In this section, we will use the ArrayField
to add an array of values to the Django model. The below example demonstrates the use of ArrayField
to create the array of TextField
.
from django.db import models
from django.contrib.postgres.fields import ArrayField
class ResearchPaper(models.Model):
paperName = models.CharField(max_length=200)
author = ArrayField(models.CharField(max_length=200), blank=True)
def __str__(self):
return self.paperName
We have created the Django model for the ResearchPaper
in the above Python code. It contains the paperName
and Authors
fields.
The paperName
field is the normal field of characters, but there can be multiple authors for a single paper, so we have used the ArrayField
to create the Authors
field.
Once we add the model in the models.py
file, we have to register the model. To register the model, create admin.py
if it doesn’t exist, and add the below code to the file.
from .models import ResearchPaper
admin.site.register(ResearchPaper)
After registering the model, users need to migrate the model to the database. For that, users need to run the below command in the terminal one by one.
python manage.py makemigrations <app_name>
python manage.py migrate
Now, we will learn to query the data from the ArrayField
according to its value.
Before we start querying the data from the ResearchPaper
table, let’s add some data.
ResearchPaper.objects.create(paperName="First paper", author=["Alice", "Bob", "jenny"])
ResearchPaper.objects.create(paperName="Second paper", author=["Alice"])
ResearchPaper.objects.create(paperName="Third paper", author=["Bob"])
ResearchPaper.objects.create(paperName="Fourth paper", author=["Alice", "Bob"])
ResearchPaper.objects.create(paperName="Fifth paper", author=["Bob", "jenny"])
So, we have added some records of research papers with different authors. Also, some papers have multiple authors, and a single author has written multiple papers.
In the below gif
, we can see that data is added to the database.
Use contains
Lookup
The contains
lookup returns all records whose lookup values are a subset of ArrayField
data.
Example Code:
ResearchPaper.objects.filter(
author__contains=["Alice"]
) # returns <QuerySet [<ResearchPaper: First paper>, <ResearchPaper: Second paper>, <ResearchPaper: Fourth paper>]>
ResearchPaper.objects.filter(
author__contains=["Alice", "Bob"]
) # returns <QuerySet [<ResearchPaper: First paper>, <ResearchPaper: Fourth paper>
Output:
Use contained_by
Lookup
The contained_by
lookup is the reverse of the contains
look-up. It returns all the records whose data is a subset of lookup values.
Example Code:
ResearchPaper.objects.filter(
author__contained_by=["Alice"]
) # returns <QuerySet [<ResearchPaper: Second paper>]>
ResearchPaper.objects.filter(
author__contained_by=["Alice", "Bob"]
) # returns <QuerySet [<ResearchPaper: Second paper>, <ResearchPaper: Third paper>, <ResearchPaper: Fourth paper>]>
Output:
Use overlap
Lookup
The overlap
lookup returns all records whose data contains any single or multiple lookup values.
Example Code:
ResearchPaper.objects.filter(
author__overlap=["Alice"]
) # returns <QuerySet [<ResearchPaper: Second paper>, <ResearchPaper: Fourth paper>]>
ResearchPaper.objects.filter(
author__overlap=["Alice", "jenny"]
) # returns <QuerySet [<ResearchPaper: Second paper>, <ResearchPaper: Fourth paper>, <ResearchPaper: Fifth paper>]>
Output:
Use len
Lookup
The len
lookup checks for the length of the data and returns all the records whose length is equivalent to the len
lookup’s value.
Example Code:
ResearchPaper.objects.filter(
author__len=1
) # returns <QuerySet [<ResearchPaper: Second paper>, <ResearchPaper: Third paper>]>
ResearchPaper.objects.filter(
author__len=3
) # returns <QuerySet [<ResearchPaper: First paper>]>
Output:
This way, we can use different lookups to query the records according to ArrayField
values.
Use ArrayField
to Create a Multi-Dimensional Array in Django Models
Users may need to create two-dimensional arrays in the Django models, and users can achieve that using the ArrayField
. Here, we have written the basic code to create a two-dimensional array in Django.
from django.db import models
from django.contrib.postgres.fields import ArrayField
class ResearchPaper(models.Model):
paperName = models.TextField()
Authors = ArrayField(
ArrayField(
models.TextField(blank=True),
)
)
In this article, we have learned to create single and multi-dimensional arrays in Django fields. Also, we have learned to use the different lookups to query the records according to values of ArrayField
.
Users can query the records for the two-dimensional array like a single-dimensional array.