How to Create Circle Button in CSS
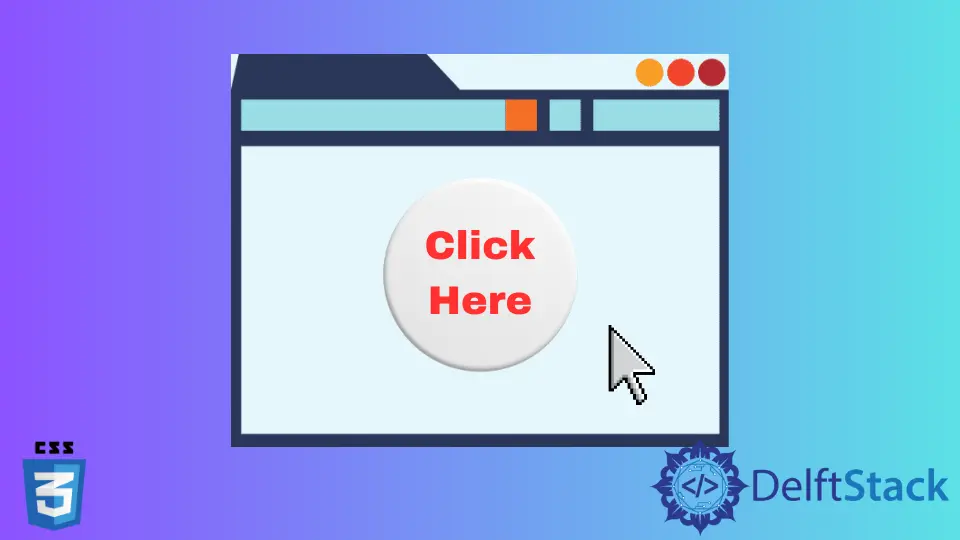
This article will introduce a method to create a circle button in CSS.
Use the border-radius
Property to Create a Circle Button in CSS
We can use the border-radius
property to create a circle button in CSS. The property creates the rounded corners to the selected element by adding the radius to the element’s corners.
The border-radius
property takes one to four values as options. We will look into the examples below.
In border-radius
with four values, the initial value applies to the top-left corner, the second one applies to the top-right corner, the third value to the bottom-right corner, and the final value to the bottom-left corner.
border-radius: 12px 50px 20px 5px
For three values, the initial value applies to the top-left corner, the second value applies to the top-right and bottom-left corners, and the final value applies to the bottom-right corner.
border-radius: 12px 50px 20px
The initial value applies to top-left and bottom-right corners in the two values, and the latter applies to top-right and bottom-left corners.
border-radius: 12px 50px
When a single radius is set, it makes a circular corner as it applies to all the corners of the element.
border-radius: 12px
We can use the border-radius
property to create a perfect circular button by providing the border-radius
as exactly half of the height and width of the button. We can use a single value, border-radius
, for the demonstration.
For example, create a button using the button
tag in HTML. Write the text Click here
inside the button.
Now in CSS, select the button and specify the height
and width
of the button to 200px
. Then, specify the border-radius
as 50%
or 100px
.
The 100px
border-radius
that we entered is half of the height and width of the button. As a result, each corner of the button will form a rounded corner with half the radius of the length of its sides.
We can use the 50%
value for border-radius
to simplify it. Thus, we built a circle button using CSS’s border-radius
property.
Example Code:
<button> Click here </button>
button {
height: 200px;
width: 200px;
border-radius: 100px;
}
button {
height: 200px;
width: 200px;
border-radius: 50%;
}
Sushant is a software engineering student and a tech enthusiast. He finds joy in writing blogs on programming and imparting his knowledge to the community.
LinkedIn