The yield Keyword in C#
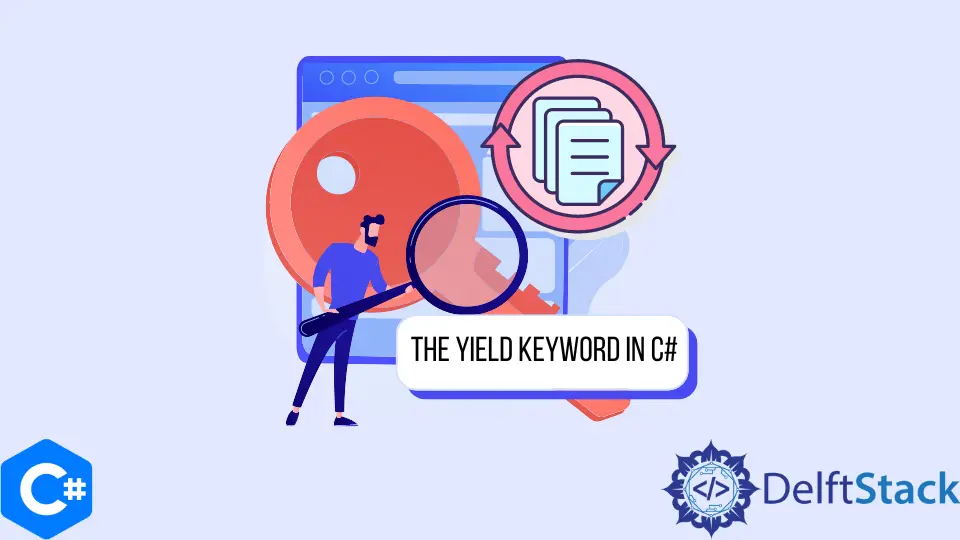
This tutorial will discuss the uses of the yield keyword in C#.
the yield
Keyword in C#
The yield
keyword is a contextual keyword in C#. It is used with the return
keyword to specify that the method it is used in is an iterator. The best use of the yield
keyword is when we are iterating through a list. With the yield
keyword, we can loop through a list and return an element from the list to the calling function and then return to the loop and start it from the next index. The yield
keyword returns an object of the IEnumerator
class. So, the return type of the function using the yield
keyword needs to be IEnumerator
. The following code example shows us how we can use the yield
keyword in C#.
using System;
using System.Collections.Generic;
namespace yield_keyword {
class Program {
static IEnumerable<string> Strings() {
List<string> Values = new List<string> { "value1", "value2", "value3", "value4" };
foreach (var val in Values) {
yield return val;
}
}
static void Main(string[] args) {
foreach (var i in Strings()) {
Console.WriteLine(i);
}
}
}
}
Output:
value1
value2
value3
value4
We created a function Strings()
that returns elements of the list of strings Values
with the yield
keyword in C#. The yield
keyword grabs a value from the Values
list, returns it to the calling function, and then continues the loop from the next iteration if the Strings()
function is invoked again.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn