How to Write to an Excel File in C#
- Setting Up Your Environment
- Writing to an Excel File Using C#
- Writing to an Existing Excel File
- Handling Excel File Exceptions
- Conclusion
- FAQ
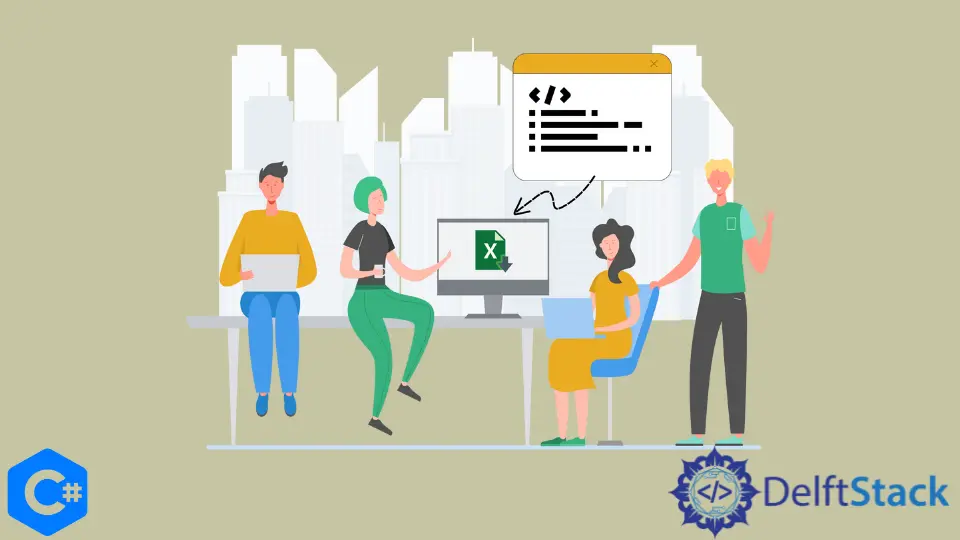
Writing to an Excel file in C# can be a straightforward task, especially when you leverage the Microsoft Office API. Whether you’re developing a desktop application or a web service, the ability to create and manipulate Excel files programmatically is invaluable. This skill can streamline reporting, automate data entry, and enhance data analysis.
In this article, we’ll explore how to write to an Excel file in C# using the Microsoft Office interop library. We’ll cover the necessary setup, provide clear code examples, and walk you through the process step by step. By the end, you’ll be equipped with the knowledge to integrate Excel file writing capabilities into your C# applications.
Setting Up Your Environment
Before diving into the code, you need to ensure that your development environment is ready. First, you’ll need to install Microsoft Office on your machine, as the interop library relies on it. Next, you will need to add a reference to the Microsoft Excel Object Library in your C# project.
To do this:
- Open your C# project in Visual Studio.
- Right-click on your project in the Solution Explorer.
- Select “Add” and then “Reference.”
- In the COM tab, find and select “Microsoft Excel XX.0 Object Library” (where XX corresponds to your version of Office).
- Click OK.
This setup allows your C# application to interact with Excel files seamlessly.
Writing to an Excel File Using C#
Now that your environment is set up, let’s look at how to write data to an Excel file using C#. Below is a simple example that demonstrates how to create a new Excel file, write some data, and save it.
using Excel = Microsoft.Office.Interop.Excel;
class Program
{
static void Main()
{
var excelApp = new Excel.Application();
excelApp.Visible = true;
var workbook = excelApp.Workbooks.Add();
var worksheet = (Excel.Worksheet)workbook.Worksheets[1];
worksheet.Cells[1, 1] = "Name";
worksheet.Cells[1, 2] = "Age";
worksheet.Cells[2, 1] = "John Doe";
worksheet.Cells[2, 2] = 30;
workbook.SaveAs(@"C:\YourPath\example.xlsx");
workbook.Close();
excelApp.Quit();
}
}
Output:
Excel file created with Name and Age columns
In this code snippet, we first create an instance of the Excel application. Setting excelApp.Visible = true
allows us to see Excel as it runs. We then add a new workbook and access the first worksheet. The Cells
property is used to write data to specific cells. Finally, we save the workbook to a specified path and close the application.
This example is a great starting point for writing to an Excel file in C#. You can modify the code to include more complex data structures, formulas, or styles as needed.
Writing to an Existing Excel File
If you want to write data to an existing Excel file rather than creating a new one, the process is quite similar. You will need to open the existing workbook and then write to the desired cells. Here’s how you can do it:
using Excel = Microsoft.Office.Interop.Excel;
class Program
{
static void Main()
{
var excelApp = new Excel.Application();
var workbook = excelApp.Workbooks.Open(@"C:\YourPath\existingFile.xlsx");
var worksheet = (Excel.Worksheet)workbook.Worksheets[1];
worksheet.Cells[2, 1] = "Jane Doe";
worksheet.Cells[2, 2] = 28;
workbook.Save();
workbook.Close();
excelApp.Quit();
}
}
Output:
Data written to existing Excel file
In this code, we open an existing Excel file using the Workbooks.Open
method. After accessing the desired worksheet, we write new data into specific cells. The Save
method is called to ensure that the changes are saved before closing the workbook and quitting the application.
This approach allows you to update existing data without overwriting the entire file, making it a flexible option for many applications.
Handling Excel File Exceptions
When working with Excel files in C#, it’s essential to handle exceptions that may arise during file operations. For instance, trying to open a file that doesn’t exist or lacking permissions can cause your application to crash. Here’s how to implement basic exception handling:
using Excel = Microsoft.Office.Interop.Excel;
class Program
{
static void Main()
{
try
{
var excelApp = new Excel.Application();
var workbook = excelApp.Workbooks.Open(@"C:\YourPath\example.xlsx");
var worksheet = (Excel.Worksheet)workbook.Worksheets[1];
worksheet.Cells[3, 1] = "Mark Smith";
worksheet.Cells[3, 2] = 35;
workbook.Save();
workbook.Close();
excelApp.Quit();
}
catch (System.Runtime.InteropServices.COMException ex)
{
Console.WriteLine("An error occurred: " + ex.Message);
}
}
}
Output:
Error message if any exception occurs
In this example, we wrap our Excel operations in a try-catch block. If a COM exception occurs, we catch it and print an error message. This simple addition can significantly improve the robustness of your application by preventing crashes and providing useful feedback.
Conclusion
Writing to an Excel file in C# using the Microsoft Office API is a powerful feature that can enhance your applications. By following the steps outlined in this article, you can easily create new Excel files, modify existing ones, and handle potential errors gracefully. Whether you are generating reports, automating data entry, or conducting data analysis, integrating Excel capabilities into your C# applications can save you time and effort. Now that you have the tools to get started, why not give it a try?
FAQ
-
What is the Microsoft Office API?
The Microsoft Office API allows developers to interact with Microsoft Office applications programmatically, enabling tasks like creating and manipulating Excel files. -
Do I need to install Microsoft Office to use the interop library?
Yes, the interop library requires Microsoft Office to be installed on your machine for it to function correctly. -
Can I write to Excel files without using Microsoft Office?
While the interop library is the most common method, there are other libraries like EPPlus and ClosedXML that allow you to manipulate Excel files without needing Microsoft Office. -
How can I handle exceptions when writing to an Excel file?
You can use try-catch blocks in your C# code to catch exceptions related to COM operations and handle them gracefully. -
Is it possible to format cells in Excel using C#?
Yes, you can format cells in Excel using properties available in the Excel interop library, such as setting font size, color, and cell borders.
using the Microsoft Office API. This comprehensive guide covers creating new files, modifying existing ones, and handling exceptions, complete with code examples and best practices. Enhance your C# applications with powerful Excel integration today.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn