How to Write a Stream to a File in C#
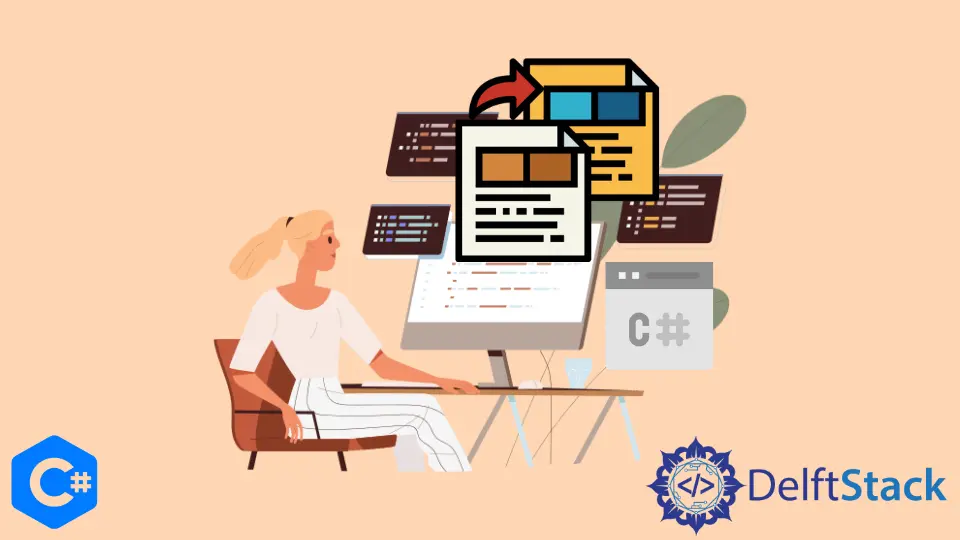
This tutorial will discuss the method to write a stream to a file in C#.
Write Stream to a File With the Stream.CopyTo()
Method in C#
The Stream.CopyTo()
method in C# copies the contents of a stream to another stream. We can open a file in the second stream and copy the input stream’s content to the output stream with the Stream.CopyTo()
method in C#.
using System;
using System.IO;
namespace read_integer {
class Program {
static void Main(string[] args) {
using (Stream inStream = File.OpenRead(@"C:\File\file.txt")) {
using (Stream outStream = File.OpenWrite(@"C:\File\file1.txt")) {
inStream.CopyTo(outStream);
}
}
}
}
}
In the above code, we write the contents of our input stream inStream
to our output stream outStream
with the inStream.CopyTo(outStream)
method in C#. We first open our input file file.txt
inside the path C:\File
to read data to the inStream
stream. After that, we open our output file file1.txt
inside the same directory C:\File
to write with the outStream
stream. We then write the contents of the inStream
to the outStream
with the Stream.CopyTo()
method.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn