How to Wait for Key Press in C#
- Understanding Console.ReadKey()
- Using Console.ReadKey() with Additional Features
- Creating a Simple Menu with Key Presses
- Conclusion
- FAQ
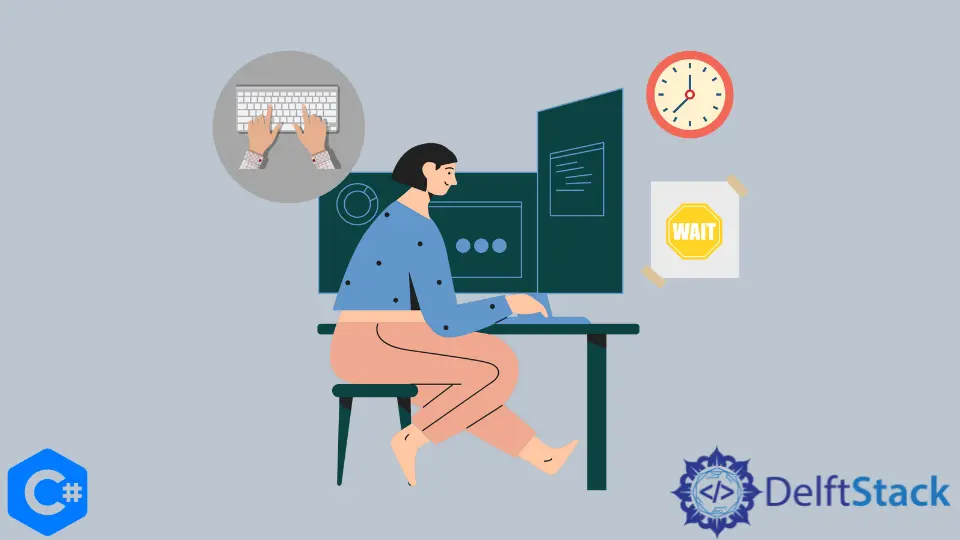
When developing applications in C#, you might find yourself needing to pause the execution of your program until the user presses a key. This is particularly useful in console applications where you want to give users a chance to read output before the program closes. The Console.ReadKey()
method is a straightforward solution for this task.
In this article, we will explore how to effectively use this method, along with practical examples and explanations. Whether you’re a beginner or an experienced developer, understanding how to implement this simple yet powerful function can enhance your C# programming skills.
Understanding Console.ReadKey()
The Console.ReadKey()
method is part of the System namespace in C#. This method reads the next key pressed by the user and returns it as a ConsoleKeyInfo object. This allows you to capture not just the character that was pressed, but also additional information like whether any modifier keys (like Shift or Ctrl) were held down.
Using Console.ReadKey()
is pretty simple. You can call it in your code wherever you want to wait for a key press. This is especially useful in console applications where the program might end immediately after displaying output, making it difficult for users to read the information.
Here’s a basic example to illustrate how it works:
using System;
class Program
{
static void Main()
{
Console.WriteLine("Press any key to continue...");
Console.ReadKey();
Console.WriteLine("Key pressed, continuing execution.");
}
}
Output:
Press any key to continue...
After the user presses a key, the output will be:
Key pressed, continuing execution.
In this example, the program first prompts the user to press any key. Once a key is pressed, it continues to execute the next line of code. This simple approach enhances user experience by preventing the console from closing immediately.
Using Console.ReadKey() with Additional Features
While the basic usage of Console.ReadKey()
is effective, you can enhance its functionality by using its parameters. The method has an overload that takes a boolean parameter to determine whether to display the pressed key in the console.
Here’s an example that demonstrates this feature:
using System;
class Program
{
static void Main()
{
Console.WriteLine("Press any key (it will not be displayed)...");
ConsoleKeyInfo keyInfo = Console.ReadKey(false);
Console.WriteLine($"\nYou pressed: {keyInfo.KeyChar}");
}
}
After pressing a key, the output will show:
You pressed: [character]
In this code, the Console.ReadKey(false)
method is called, which means the pressed key will not be displayed in the console. After the key press, the program informs the user of which key was pressed. This can be useful in scenarios where you want to capture input without cluttering the console output.
Creating a Simple Menu with Key Presses
You can also use Console.ReadKey()
to create interactive menus in your console applications. This allows users to navigate through options by pressing specific keys. Here’s a simple example:
using System;
class Program
{
static void Main()
{
Console.WriteLine("Menu:");
Console.WriteLine("1. Option 1");
Console.WriteLine("2. Option 2");
Console.WriteLine("Press 'q' to quit.");
while (true)
{
ConsoleKeyInfo keyInfo = Console.ReadKey(true);
if (keyInfo.Key == ConsoleKey.D1)
{
Console.WriteLine("You selected Option 1.");
}
else if (keyInfo.Key == ConsoleKey.D2)
{
Console.WriteLine("You selected Option 2.");
}
else if (keyInfo.Key == ConsoleKey.Q)
{
Console.WriteLine("Exiting...");
break;
}
}
}
}
Output:
Menu:
1. Option 1
2. Option 2
Press 'q' to quit.
When the user presses ‘1’, ‘2’, or ‘q’, the output will change accordingly:
You selected Option 1.
or
You selected Option 2.
or
Exiting...
In this example, a simple menu is presented to the user. The program continuously waits for a key press and responds accordingly. This creates a more interactive experience, allowing users to make selections without needing to enter commands manually.
Conclusion
In this article, we explored how to wait for a key press in C# using the Console.ReadKey()
method. We discussed its basic usage, various features, and how it can be applied to create interactive console menus. Mastering this method can significantly improve user interaction in your console applications. Whether you’re building a simple program or a more complex application, knowing how to effectively wait for user input is an essential skill in C# programming.
FAQ
-
What does the Console.ReadKey() method do?
Console.ReadKey() waits for the user to press a key and returns information about that key. -
Can I suppress the display of the pressed key in the console?
Yes, by using Console.ReadKey(false), you can prevent the pressed key from being displayed. -
Is Console.ReadKey() suitable for GUI applications?
Console.ReadKey() is primarily designed for console applications and is not suitable for GUI applications. -
How can I create a menu using Console.ReadKey()?
You can create a loop that waits for key presses and responds based on the pressed key to navigate through menu options. -
Can I capture special keys with Console.ReadKey()?
Yes, Console.ReadKey() allows you to capture special keys like arrow keys, function keys, and modifier keys.
using the Console.ReadKey() method. This article covers its basic usage, enhanced features, and practical examples. Improve user interaction in your console applications by mastering this essential skill.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn