typedef Equivalent in C#
- Understanding the Need for Type Aliases
-
Using the
using
Directive - Creating Structs for Complex Types
- Utilizing Classes for Type Abstraction
- Conclusion
- FAQ
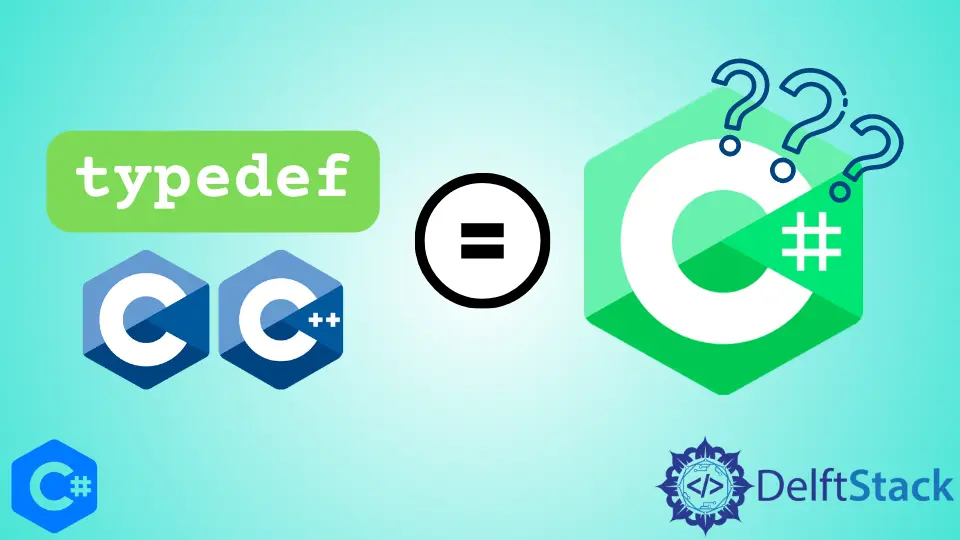
When transitioning from C or C++ to C#, programmers often seek the familiar typedef keyword, which allows for the creation of type aliases. Unfortunately, C# doesn’t have a direct equivalent to typedef. However, C# provides other ways to achieve similar functionality through features like using
, class
, and struct
. Understanding these alternatives can significantly enhance code readability and maintainability.
In this article, we’ll explore how to create type aliases and simplify complex types in C#. We’ll also provide practical examples and explanations to help you grasp these concepts effectively.
Understanding the Need for Type Aliases
In C and C++, typedef is commonly used to define new names for existing types, making the code cleaner and easier to read. For instance, if you frequently use a complex structure, creating a typedef can simplify your code significantly. In C#, while there isn’t a direct typedef keyword, you can still achieve similar results using different constructs. This is essential for developers who want to maintain clarity in their code, especially when dealing with complex types or generics.
Using the using
Directive
One of the most straightforward ways to create type aliases in C# is through the using
directive. This allows you to define a shorthand for a namespace or a type, making your code cleaner and more manageable. Here’s how you can use it:
using MyInt = System.Int32;
class Program
{
static void Main()
{
MyInt number = 42;
Console.WriteLine(number);
}
}
Output:
42
In this example, we define MyInt
as an alias for System.Int32
. When you declare a variable of type MyInt
, it is essentially the same as declaring a variable of type int
. This approach is particularly useful when working with long or complex type names, as it reduces the amount of code you need to write and enhances readability.
Additionally, using the using
directive can help avoid name clashes when working with multiple namespaces. By creating an alias, you can ensure that your code remains clear and unambiguous, which is vital in large projects.
Creating Structs for Complex Types
Another method to create type aliases in C# is by defining a struct
. This is particularly useful for grouping related data together. Structs can encapsulate multiple fields and methods, providing a clear structure that can be easily referenced throughout your code. Here’s an example:
struct Point
{
public int X;
public int Y;
public Point(int x, int y)
{
X = x;
Y = y;
}
}
class Program
{
static void Main()
{
Point p = new Point(10, 20);
Console.WriteLine($"X: {p.X}, Y: {p.Y}");
}
}
Output:
X: 10, Y: 20
In this code, we define a Point
struct that holds two integer values, X
and Y
. By creating a struct, we encapsulate related data, making it easier to manage. When you create an instance of Point
, you can access its properties directly, which improves code clarity and organization.
Structs in C# are value types, meaning they are stored on the stack, making them efficient for performance-critical applications. However, it’s essential to note that structs should be used for small data structures, as large structs can lead to performance issues.
Utilizing Classes for Type Abstraction
If you need more functionality than what a struct can provide, consider using classes. Classes in C# allow you to create complex types with methods, properties, and events. This can be particularly helpful when you want to encapsulate behavior along with data. Here’s an example:
class Circle
{
public double Radius { get; set; }
public Circle(double radius)
{
Radius = radius;
}
public double Area()
{
return Math.PI * Radius * Radius;
}
}
class Program
{
static void Main()
{
Circle circle = new Circle(5);
Console.WriteLine($"Area of circle: {circle.Area()}");
}
}
Output:
Area of circle: 78.53981633974483
In this example, we define a Circle
class that holds a radius and a method to calculate its area. Classes provide a more extensive feature set than structs, including inheritance and polymorphism, which can be beneficial in larger applications. By utilizing classes, you can create a type that not only holds data but also encapsulates behavior, leading to more organized and maintainable code.
Conclusion
While C# does not have a direct equivalent to the typedef keyword found in C and C++, it offers several alternatives to create type aliases and improve code readability. By using the using
directive, structs, and classes, developers can effectively manage complex types and enhance their code’s clarity. Understanding these constructs is essential for any C# programmer looking to write clean, maintainable, and efficient code. Embrace these alternatives, and you’ll find your C# programming experience more enjoyable and productive.
FAQ
-
What is the purpose of typedef in C and C++?
typedef is used to create type aliases, simplifying code and improving readability. -
Can I use typedef in C#?
No, C# does not have a typedef keyword, but you can use alternatives like using directives, structs, and classes. -
How does the using directive work in C#?
The using directive allows you to create a shorthand for a type or namespace, making your code cleaner and more manageable. -
When should I use structs instead of classes in C#?
Use structs for small data structures that hold related data, while classes are better for more complex types with behavior. -
Are there any performance differences between structs and classes in C#?
Yes, structs are value types stored on the stack, making them more efficient for small data. Classes are reference types stored on the heap, which can impact performance in certain scenarios.
and learn how to create type aliases using the using directive, structs, and classes. This article provides practical examples and detailed explanations to help you enhance your C# programming skills and improve code readability. Discover how to manage complex types effectively in your projects.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn