Timer in C#
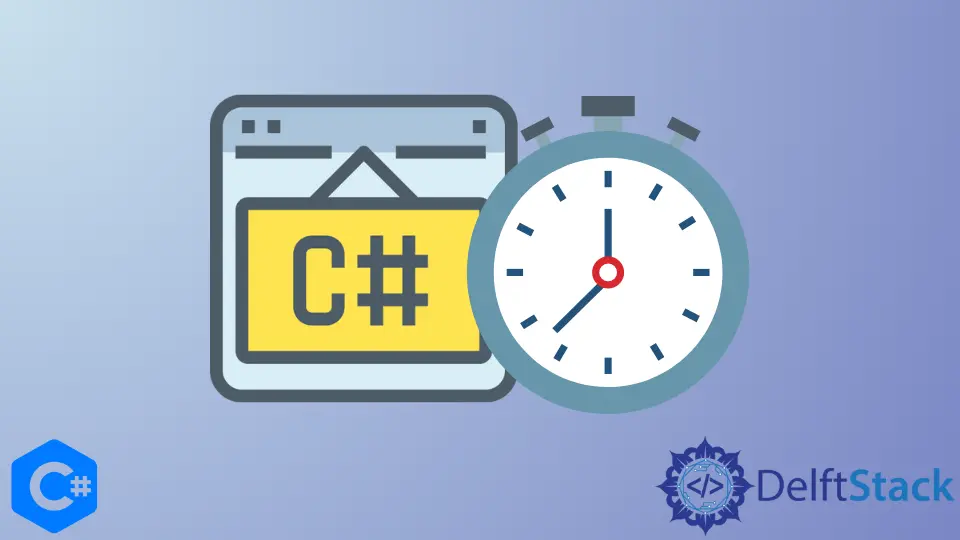
This tutorial will discuss the methods for creating a timer in C#.
Create a Timer With the Timer
Class in C#
The Timer
class is used to create an event after a set time interval in C#. We can generate an event that repeats itself after a specified amount of time with the Timer
class in C#. The Timer.Elapsed
event is used to specify the event to be repeated. The following code example shows us how to create a timer that repeats an event after a specified interval of time with the Timer
class in C#.
using System;
using System.Timers;
namespace timer {
class Program {
private static void OnTimedEvent(object source, ElapsedEventArgs e) {
Console.WriteLine("Hello World!");
}
static void Main(string[] args) {
System.Timers.Timer myTimer = new System.Timers.Timer();
myTimer.Elapsed += new ElapsedEventHandler(OnTimedEvent);
myTimer.Interval = 500;
myTimer.Enabled = true;
Console.WriteLine("Press \'e\' to escape the sample.");
while (Console.Read() != 'e')
;
}
}
}
Output:
Press 'e' to escape the sample.
Hello World!
e
In the above code, we created a timer that repeats the output Hello World!
after every 500
milliseconds until the character e
is entered in C#. We first initialized the instance myTimer
of the Timer
class. Then we specified the OnTimedEvent()
function to be the event for the Time.Elapsed
event. The interval is set to be 500
milliseconds with the myTimer.Interval
property. In the end, we started the timer with the myTimer.Enabled
property.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn