How to Use Strings in Switch Statement in C#
Minahil Noor
Feb 02, 2024
Csharp
Csharp String
Csharp Switch
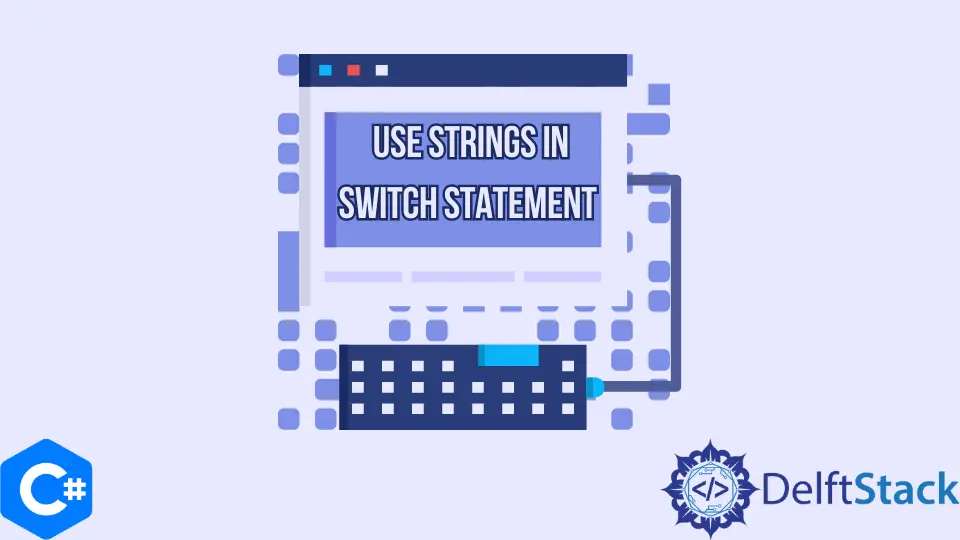
This article will introduce a method to use the string in the switch statement in C#.
Use Strings in the switch
Statement in C#
There is no special method of using strings in the switch statement. We can simply create cases by assigning the value in double-quotes that represents a string.
The program below shows how we can use strings in the switch statement in C#.
using System;
class StringinSwitch {
static public void Main() {
string mystring = "Rose";
switch (mystring) {
case "Jasmine":
Console.WriteLine("The flower is Jasmine");
break;
case "Lili":
Console.WriteLine("The flower is Lili");
break;
case "Rose":
Console.WriteLine("The flower is Rose");
break;
case "Hibiscus":
Console.WriteLine("The flower is Hibiscus");
break;
case "Daisy":
Console.WriteLine("The flower is Daisy");
break;
default:
Console.WriteLine("No Flower Selected");
break;
}
}
}
Output:
The flower is Rose
We have passed the string in the switch statement. The switch
statement has returned the value according to the value to the given string.
If we pass a string that is not in the cases, then the switch
statement will use the default case
.
using System;
class StringinSwitch {
static public void Main() {
string mystring = "Sun Flower";
switch (mystring) {
case "Jasmine":
Console.WriteLine("The flower is Jasmine");
break;
case "Lili":
Console.WriteLine("The flower is Lili");
break;
case "Rose":
Console.WriteLine("The flower is Rose");
break;
case "Hibiscus":
Console.WriteLine("The flower is Hibiscus");
break;
case "Daisy":
Console.WriteLine("The flower is Daisy");
break;
default:
Console.WriteLine("No Flower Selected");
break;
}
}
}
Output:
No Flower Selected
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Related Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#