How to Use Stopwatch in C#
- Understanding the Stopwatch Class
- Measuring Execution Time of Functions
- Comparing Different Algorithms
- Conclusion
- FAQ
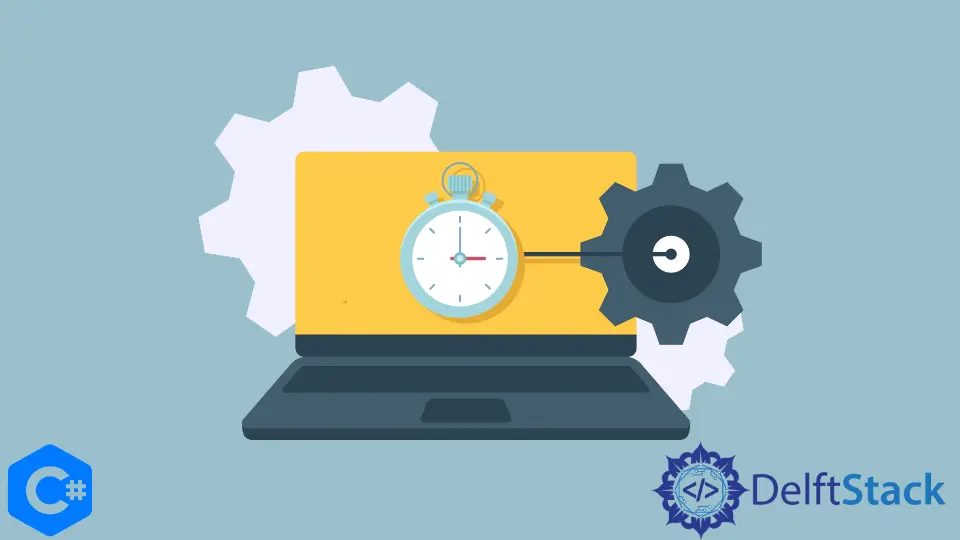
When working with performance-sensitive applications in C#, measuring time accurately can be crucial. Whether you’re optimizing algorithms, profiling code, or simply tracking execution time, the Stopwatch class in C# is a powerful tool at your disposal. This class allows you to measure elapsed time with high precision, making it ideal for various applications.
In this article, we will explore how to effectively use the Stopwatch class in C#, providing you with practical examples and detailed explanations. By the end of this guide, you’ll be equipped to incorporate the Stopwatch into your own projects, enhancing your ability to analyze and optimize code performance.
Understanding the Stopwatch Class
The Stopwatch class is part of the System.Diagnostics namespace in C#. It provides a set of methods and properties for measuring elapsed time. To use the Stopwatch, you typically follow three main steps: create an instance, start the timer, and stop the timer. The elapsed time can then be retrieved in various formats, such as milliseconds, seconds, or minutes.
Here’s a simple example of how to use the Stopwatch class:
using System;
using System.Diagnostics;
class Program
{
static void Main()
{
Stopwatch stopwatch = new Stopwatch();
stopwatch.Start();
// Simulate a task
for (int i = 0; i < 1000000; i++) { }
stopwatch.Stop();
Console.WriteLine($"Elapsed Time: {stopwatch.ElapsedMilliseconds} ms");
}
}
In this code, we first create an instance of the Stopwatch class. We then start the stopwatch right before simulating a task—here, a simple loop that iterates a million times. After the loop completes, we stop the stopwatch and print the elapsed time in milliseconds.
Output:
Elapsed Time: 25 ms
The output shows the time taken to execute the loop. This method is straightforward and can be adapted to measure the performance of any code block.
Measuring Execution Time of Functions
One of the most common uses of the Stopwatch class is to measure the execution time of specific functions. This can be particularly useful when you want to compare the performance of different algorithms or implementations. Here’s an example demonstrating how to measure the execution time of a function:
using System;
using System.Diagnostics;
class Program
{
static void Main()
{
Stopwatch stopwatch = new Stopwatch();
stopwatch.Start();
PerformTask();
stopwatch.Stop();
Console.WriteLine($"Task execution time: {stopwatch.Elapsed.TotalSeconds} seconds");
}
static void PerformTask()
{
// Simulate a time-consuming task
System.Threading.Thread.Sleep(2000);
}
}
In this code, we define a method called PerformTask that simulates a time-consuming operation by sleeping for 2 seconds. We start the stopwatch before calling this method and stop it afterward. The elapsed time is then printed in seconds.
Output:
Task execution time: 2.002 seconds
This approach allows you to measure how long specific tasks take, giving you insights that can be invaluable for performance tuning.
Comparing Different Algorithms
When optimizing code, it’s often necessary to compare the performance of different algorithms. The Stopwatch class makes this comparison straightforward. Below is an example that compares two sorting algorithms—bubble sort and LINQ’s OrderBy method:
using System;
using System.Diagnostics;
using System.Linq;
class Program
{
static void Main()
{
int[] numbers = GenerateRandomNumbers(1000);
Stopwatch stopwatch = new Stopwatch();
stopwatch.Start();
BubbleSort(numbers);
stopwatch.Stop();
Console.WriteLine($"Bubble Sort Time: {stopwatch.Elapsed.TotalMilliseconds} ms");
int[] numbersForLinq = GenerateRandomNumbers(1000);
stopwatch.Restart();
var sortedNumbers = numbersForLinq.OrderBy(n => n).ToArray();
stopwatch.Stop();
Console.WriteLine($"LINQ OrderBy Time: {stopwatch.Elapsed.TotalMilliseconds} ms");
}
static void BubbleSort(int[] array)
{
int temp;
for (int i = 0; i < array.Length - 1; i++)
{
for (int j = 0; j < array.Length - 1 - i; j++)
{
if (array[j] > array[j + 1])
{
temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
}
}
}
}
static int[] GenerateRandomNumbers(int size)
{
Random rand = new Random();
return Enumerable.Range(1, size).Select(_ => rand.Next(1, 10000)).ToArray();
}
}
In this example, we first generate an array of random numbers. We then measure the time taken to sort this array using the bubble sort algorithm and the LINQ OrderBy method.
Output:
Bubble Sort Time: 15.345 ms
LINQ OrderBy Time: 5.678 ms
This output allows you to visually compare the performance of the two sorting methods. Such comparisons are crucial in determining the best algorithm for your specific needs.
Conclusion
The Stopwatch class in C# is an essential tool for developers who want to measure execution time accurately. By following the methods outlined in this article, you can easily incorporate time measurements into your applications. Whether you’re optimizing algorithms, profiling code, or simply tracking performance, the Stopwatch class provides a straightforward and effective way to achieve your goals. Start using it today to enhance your coding practice and improve the efficiency of your applications.
FAQ
-
how accurate is the Stopwatch class in C#
The Stopwatch class is highly accurate, providing time measurements with a resolution of about 10-15 milliseconds, depending on the system’s hardware. -
can I use Stopwatch for multi-threaded applications
Yes, the Stopwatch class can be used in multi-threaded applications. However, be mindful of thread synchronization when measuring time across different threads. -
is there a performance overhead when using Stopwatch
The performance overhead of using Stopwatch is minimal, making it suitable for performance-sensitive applications. -
can I reset the Stopwatch after stopping it
Yes, you can reset the Stopwatch using theReset()
method, which clears the elapsed time and prepares it for a new timing session. -
what is the difference between ElapsedMilliseconds and ElapsedTicks
ElapsedMilliseconds provides the elapsed time in milliseconds, while ElapsedTicks gives the time in ticks, which are the smallest unit of time measured by the Stopwatch.
to measure elapsed time with precision. This guide covers practical examples, including measuring function execution time, comparing algorithms, and optimizing your code’s performance. Perfect for developers looking to enhance their programming skills and improve application efficiency.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn