How to Sort an Array in C#
- Understanding Array.Sort() Method
- Sorting an Array of Strings
- Sorting with Custom Comparisons
- Sorting an Array of Objects
- Conclusion
- FAQ
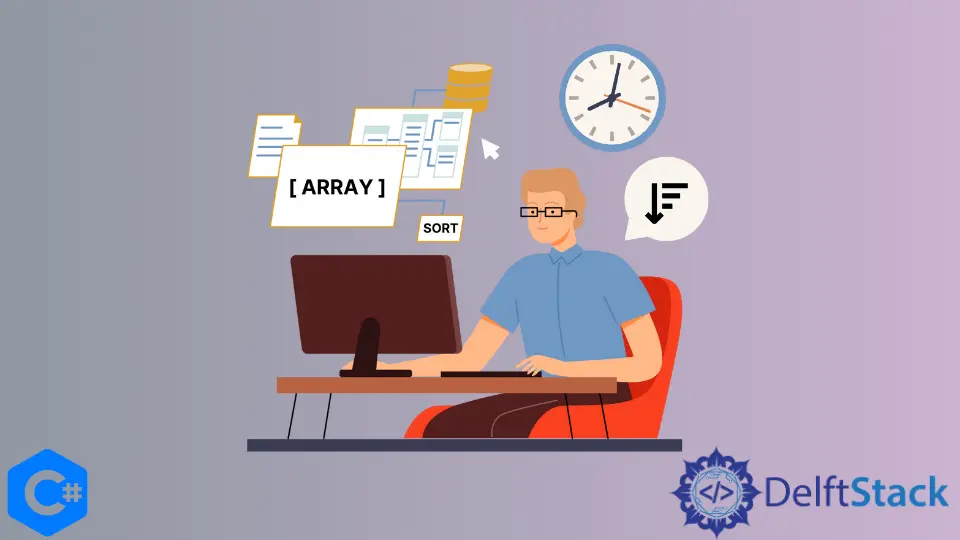
Sorting an array is a fundamental task in programming that allows you to organize data effectively. In C#, one of the most straightforward ways to sort an array is by using the built-in Array.Sort()
method. This method is efficient and easy to implement, making it a popular choice among developers. Whether you’re dealing with a simple list of integers or a complex array of objects, understanding how to sort arrays in C# can significantly enhance your programming skills.
In this article, we will explore the Array.Sort()
method in detail, providing clear examples and explanations to help you master array sorting in C#.
Understanding Array.Sort() Method
The Array.Sort()
method is part of the System namespace in C#. This method sorts the elements in a one-dimensional array. The sorting is done in ascending order by default, which means that smaller elements will appear before larger ones. It can handle arrays of any data type, including integers, strings, and custom objects.
Here’s a simple example demonstrating how to use the Array.Sort()
method to sort an array of integers:
using System;
class Program
{
static void Main()
{
int[] numbers = { 5, 3, 8, 1, 2 };
Array.Sort(numbers);
foreach (int number in numbers)
{
Console.WriteLine(number);
}
}
}
Output:
1
2
3
5
8
In this code, we create an integer array called numbers
and populate it with unsorted values. We then call Array.Sort(numbers)
, which sorts the array in ascending order. Finally, we use a foreach
loop to print the sorted numbers. The result is a neatly organized array, showcasing the power of the Array.Sort()
method.
Sorting an Array of Strings
Sorting arrays is not limited to numerical values; you can also sort arrays of strings. The Array.Sort()
method works seamlessly with string arrays as well. Here’s a quick example:
using System;
class Program
{
static void Main()
{
string[] fruits = { "Banana", "Apple", "Cherry", "Mango" };
Array.Sort(fruits);
foreach (string fruit in fruits)
{
Console.WriteLine(fruit);
}
}
}
Output:
Apple
Banana
Cherry
Mango
In this example, we define an array of fruit names. By calling Array.Sort(fruits)
, we sort the array alphabetically. The result is a neatly arranged list of fruit names, demonstrating how versatile the Array.Sort()
method is for different data types.
Sorting with Custom Comparisons
Sometimes, you may need to sort an array based on specific criteria. The Array.Sort()
method allows for custom sorting using a comparison delegate. Here’s how you can implement it:
using System;
class Program
{
static void Main()
{
string[] names = { "John", "Alice", "Bob", "Charlie" };
Array.Sort(names, (x, y) => y.CompareTo(x));
foreach (string name in names)
{
Console.WriteLine(name);
}
}
}
Output:
John
Charlie
Bob
Alice
In this code, we sort the names
array in descending order. By using a lambda expression as a comparison delegate, we specify the sorting criteria. The CompareTo
method is called on each pair of elements, allowing us to customize how the sorting is performed. This flexibility makes Array.Sort()
a powerful tool in your C# programming arsenal.
Sorting an Array of Objects
Sorting arrays of objects is another common scenario. To sort an array of custom objects, you need to implement the IComparable
interface in your class. Here’s an example:
using System;
class Person : IComparable<Person>
{
public string Name { get; set; }
public int Age { get; set; }
public int CompareTo(Person other)
{
return this.Age.CompareTo(other.Age);
}
}
class Program
{
static void Main()
{
Person[] people = {
new Person { Name = "John", Age = 25 },
new Person { Name = "Alice", Age = 30 },
new Person { Name = "Bob", Age = 20 }
};
Array.Sort(people);
foreach (Person person in people)
{
Console.WriteLine($"{person.Name} - {person.Age}");
}
}
}
Output:
Bob - 20
John - 25
Alice - 30
In this example, we define a Person
class that implements IComparable<Person>
. The CompareTo
method is overridden to sort the Person
objects by their age. When we call Array.Sort(people)
, it sorts the array based on the age property. This approach allows you to sort complex data structures efficiently, demonstrating the versatility of the Array.Sort()
method.
Conclusion
Sorting arrays in C# is a straightforward task thanks to the Array.Sort()
method. Whether you are working with integers, strings, or custom objects, this method provides a flexible and efficient way to organize your data. By understanding how to implement basic sorting and custom comparisons, you can enhance your coding skills and improve the functionality of your applications. Start practicing with these examples, and soon you’ll be sorting arrays like a pro!
FAQ
-
How does the Array.Sort() method work in C#?
The Array.Sort() method sorts the elements of an array in ascending order by default. It can handle various data types and uses an efficient sorting algorithm. -
Can I sort an array in descending order using Array.Sort()?
Yes, you can sort an array in descending order by providing a custom comparison delegate to the Array.Sort() method. -
What if I want to sort an array of custom objects?
To sort an array of custom objects, implement the IComparable interface in your class and override the CompareTo method to define the sorting criteria. -
Is Array.Sort() efficient for large datasets?
Yes, Array.Sort() is optimized for performance and can handle large datasets efficiently, making it suitable for most sorting tasks in C#. -
Can I sort multidimensional arrays with Array.Sort()?
No, the Array.Sort() method only works with one-dimensional arrays. You will need to implement custom sorting logic for multidimensional arrays.
#. It includes the Array.Sort() method, providing clear examples and explanations for sorting integers, strings, and custom objects. Learn how to implement sorting efficiently in your C# applications and enhance your programming skills.