Singleton Class in C#
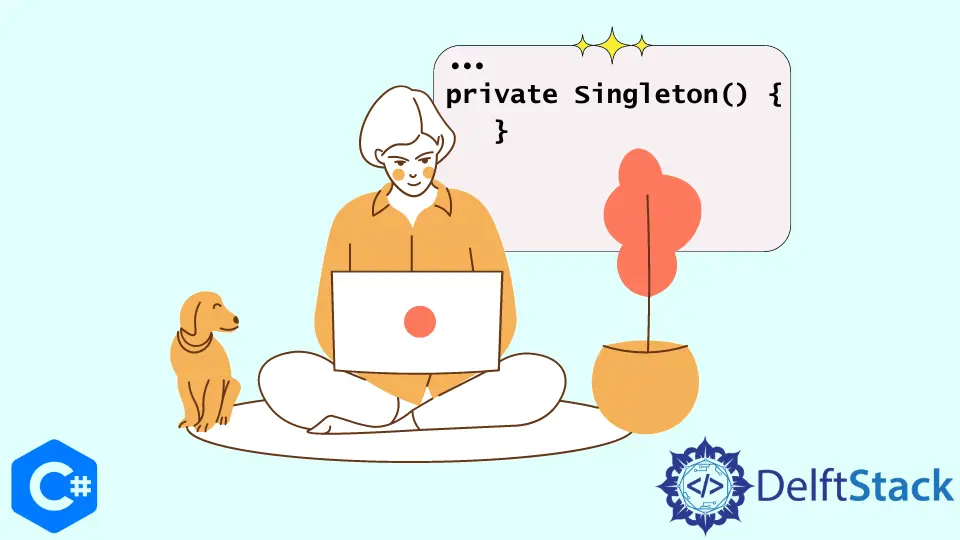
This tutorial will discuss the properties of a Singleton class in C#.
Singleton Class in C#
A singleton class only allows a single instance of itself to be created and gives easy access to that instance. Typically we cannot specify any parameters while initializing an instance of a singleton class. The instances of the singleton classes must be initialized lazily. It means that an instance must only be initialized when it is first needed. The following code example shows us how we can create a basic singleton class in C#.
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton Instance {
get {
if (instance == null)
instance = new Singleton();
return instance;
}
}
}
In the above singleton class Singleton
, we declared an instance of the class instance
that contains a reference to the only instance of the Singleton
class. We also defined a private constructor Singleton
and the property Instance
that initializes the value of instance
.
Normally, it is never advisable to use the singleton pattern in C#. It is because, no matter what our situation is, there is always a better and more elegant solution or approach available in C#. The singleton pattern is one of those things that we should be aware of but never use in our applications.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn