How to Serialize an Object to XML in C#
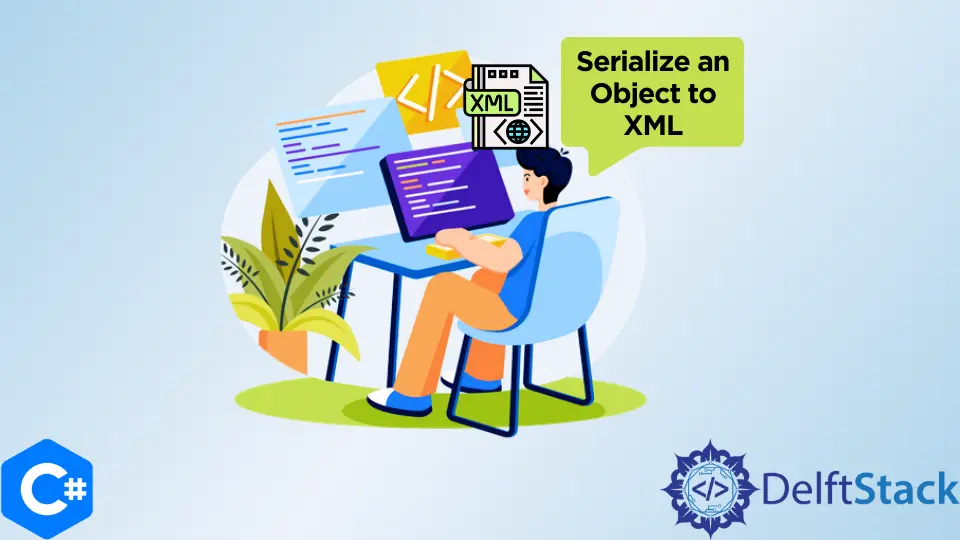
This tutorial will discuss how to serialize a class object to an XML file or string in C#.
Serialize Object to XML With the XmlSerializer
Class in C#
Serializing means converting class objects to XML or binary format. The XmlSerializer
class converts class objects to XML and vice versa in C#. The XmlSerializer.Serialize()
method converts all the public fields and properties of a class object to XML format. We need to define our class with the public
access specifier if we want to write it to an XML file or string. The following code example shows us how to serialize a class object to an XML string variable with the XmlSerializer
class in C#.
using System;
using System.IO;
using System.Linq;
using System.Xml;
using System.Xml.Serialization;
namespace serialize_object {
public class Sample {
public string value { get; set; }
}
class Program {
static void Main(string[] args) {
Sample obj = new Sample();
obj.value = "this is some value";
string xml = "";
XmlSerializer serializer = new XmlSerializer(typeof(Sample));
using (var sww = new StringWriter()) {
using (XmlWriter writer = XmlWriter.Create(sww)) {
serializer.Serialize(writer, obj);
xml = sww.ToString(); // Your XML
}
}
Console.WriteLine(xml);
}
}
}
Output:
<?xml version="1.0" encoding="utf-16"?><Sample xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="https://www.w3.org/2001/XMLSchema"><value>this is some value</value></Sample>
In the above code, we serialized the object obj
of the class Sample
to an XML string variable xml
with the XmlSerializer.Serialize()
function in C#. We first initialized an instance of the XmlSerializer
class by passing the typeof(Sample)
parameter. This creates an instance of the XmlSerializer
class of type Sample
. We then created an instance of the StringWriter
class to write data into a string variable. We then created an instance of the XmlWriter
class to write to the instance of the StringWriter
class. We then wrote the object to the instance of the StringWriter
class with serializer.Serialize(writer, obj)
and stored the outcome to the string variable xml
with the ToString()
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn