How to Run a Command-Prompt Command in C#
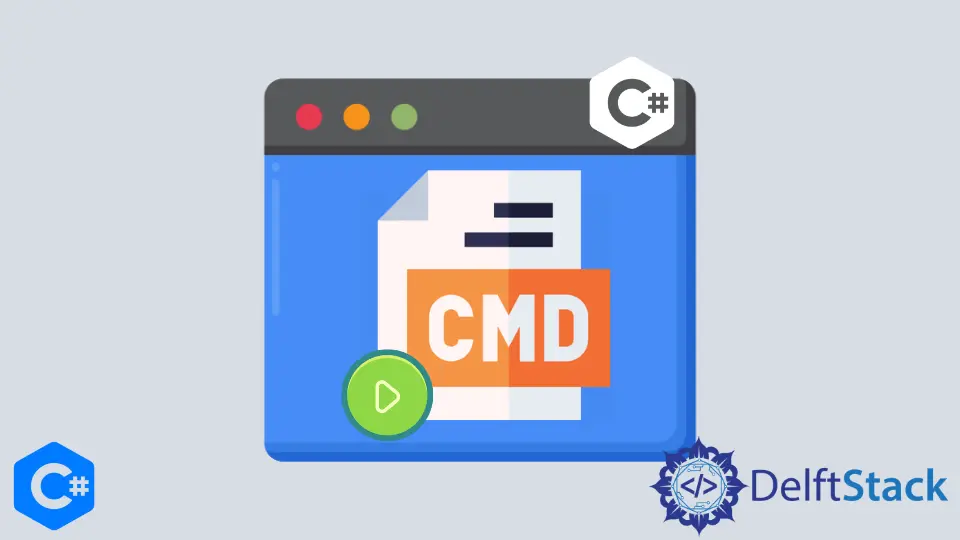
This tutorial will discuss methods to run a Command-Prompt command in C#.
Run a Command-Prompt Command With the Process.Start()
Function in C#
The Process
class provides functionality to start and stop processes in C#. The Process.Start()
function is used to start a process in C#. We can pass cmd.exe
and the command as parameters to the Process.Start()
function to start command-prompt with the specified command. The following code example shows us how we can run a command-prompt command with the Process.Start()
function in C#.
using System.Diagnostics;
namespace run_cmd_command {
class Program {
static void Main(string[] args) {
string command = "/C notepad.exe";
Process.Start("cmd.exe", command);
}
}
}
We started the command-prompt cmd.exe
and ran the command to open the notepad notepad.exe
with the Process.Start()
function in C#. The command string command
has to start with /C
for this method to work.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn