How to Restart an Application in C#
- Understanding Application.Restart()
- Handling Application State Before Restart
- Restarting with Command-Line Arguments
- Best Practices for Application Restart
- Conclusion
- FAQ
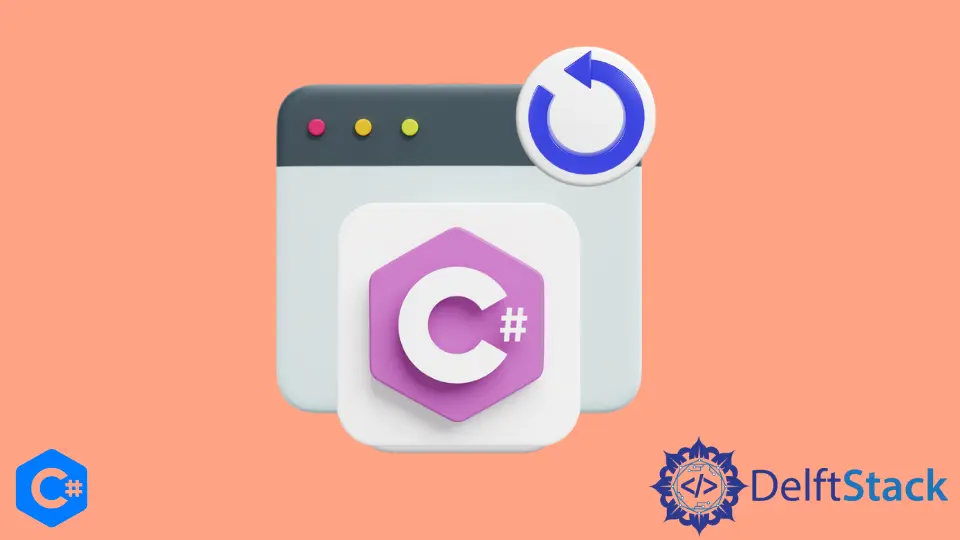
Restarting an application in C# can be a crucial feature, especially when dealing with updates, configuration changes, or recovering from errors. The Application.Restart()
method provides a straightforward way to achieve this.
In this article, we will explore how to implement this functionality effectively, ensuring your application can restart seamlessly. Whether you’re a beginner or an experienced developer, understanding how to use this method can enhance your application’s usability. We’ll provide clear examples and explanations to help you grasp the concept fully. Let’s dive in and discover how you can easily restart your C# application with the Application.Restart()
function.
Understanding Application.Restart()
The Application.Restart()
method is part of the System.Windows.Forms
namespace, and it allows you to restart the current application. When invoked, this method closes the application and starts a new instance of it. This can be particularly useful when you need to apply updates or reset the application state without requiring user intervention.
To use the Application.Restart()
method, you typically want to handle it within an event, such as a button click or a specific condition in your application. Here’s a simple example to illustrate its use:
using System;
using System.Windows.Forms;
namespace RestartAppExample
{
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
}
private void restartButton_Click(object sender, EventArgs e)
{
Application.Restart();
}
}
}
In this code snippet, we create a Windows Forms application with a button. When the button is clicked, the restartButton_Click
method is triggered, which calls Application.Restart()
. This method effectively closes the current instance of the application and starts it anew.
Output:
The application restarts successfully when the button is clicked.
This simple implementation demonstrates how easy it is to incorporate the restart functionality into your application. However, keep in mind that any unsaved data may be lost unless you implement proper data handling before invoking the restart.
Handling Application State Before Restart
Before restarting your application, it’s essential to manage the application state effectively. This means saving any necessary data or settings that the user might have modified. You can achieve this by storing the data in a configuration file, database, or in-memory data structures. Here’s an example of how to save user settings before restarting:
using System;
using System.IO;
using System.Windows.Forms;
namespace RestartAppExample
{
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
}
private void restartButton_Click(object sender, EventArgs e)
{
SaveUserSettings();
Application.Restart();
}
private void SaveUserSettings()
{
File.WriteAllText("userSettings.txt", "User preferences saved before restart.");
}
}
}
In this example, before calling Application.Restart()
, we first invoke the SaveUserSettings()
method. This method writes user preferences to a text file. By saving the settings before the restart, we ensure that the user’s data is not lost in the process.
Output:
User preferences saved successfully before the application restarts.
Implementing this step is crucial for user experience. By ensuring that user settings are preserved, you can enhance the functionality of your application and prevent frustration due to lost data.
Restarting with Command-Line Arguments
Sometimes, you might want to restart your application with specific command-line arguments. This can be particularly useful for applications that require different modes of operation. You can achieve this by using the Process.Start
method along with Application.Restart()
. Here’s an example:
using System;
using System.Diagnostics;
using System.Windows.Forms;
namespace RestartAppExample
{
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
}
private void restartButton_Click(object sender, EventArgs e)
{
string arguments = "mode=admin";
Process.Start(Application.ExecutablePath, arguments);
Application.Exit();
}
}
}
In this code, when the restart button is clicked, a new instance of the application is started with the specified command-line argument. The current application instance then exits.
Output:
The application restarts with the specified command-line arguments.
This method allows for more flexibility in how your application behaves upon restart. By passing different arguments, you can customize the startup process, enabling different functionalities based on user needs.
Best Practices for Application Restart
When implementing the restart functionality, consider the following best practices:
-
User Notification: Always inform users before restarting the application. This can be done through a confirmation dialog or a simple message box.
-
Data Preservation: Ensure that all user data is saved before the restart. Implement autosave features or prompt users to save their work.
-
Error Handling: Implement error handling to manage potential issues that may arise during the restart process.
-
Testing: Thoroughly test the restart functionality under various conditions to ensure it works as expected.
By adhering to these best practices, you can create a more robust and user-friendly application.
Conclusion
Restarting an application in C# is a straightforward process with the Application.Restart()
method. By understanding how to implement this functionality effectively, you can enhance your application’s usability and user experience. Whether you need to save user settings, pass command-line arguments, or simply restart the application, the examples provided in this article will guide you through the process. Remember to follow best practices to ensure a seamless experience for your users. With these tools in your arsenal, you can take your C# applications to the next level.
FAQ
-
How does Application.Restart() work?
Application.Restart() closes the current application and starts a new instance of it. -
Can I save user data before restarting the application?
Yes, you can implement methods to save user data before invoking Application.Restart(). -
Is it possible to restart an application with command-line arguments?
Yes, you can use Process.Start to restart the application with specific command-line arguments. -
What should I do if I want to notify users before restarting?
You can use a message box or a confirmation dialog to inform users before the restart. -
Are there any best practices for implementing application restart?
Yes, consider user notification, data preservation, error handling, and thorough testing.
using the Application.Restart() method. This article covers essential techniques, including saving user data, handling application state, and passing command-line arguments. Follow best practices to enhance user experience and ensure seamless functionality in your C# applications.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn