How to Read a JSON File in C#
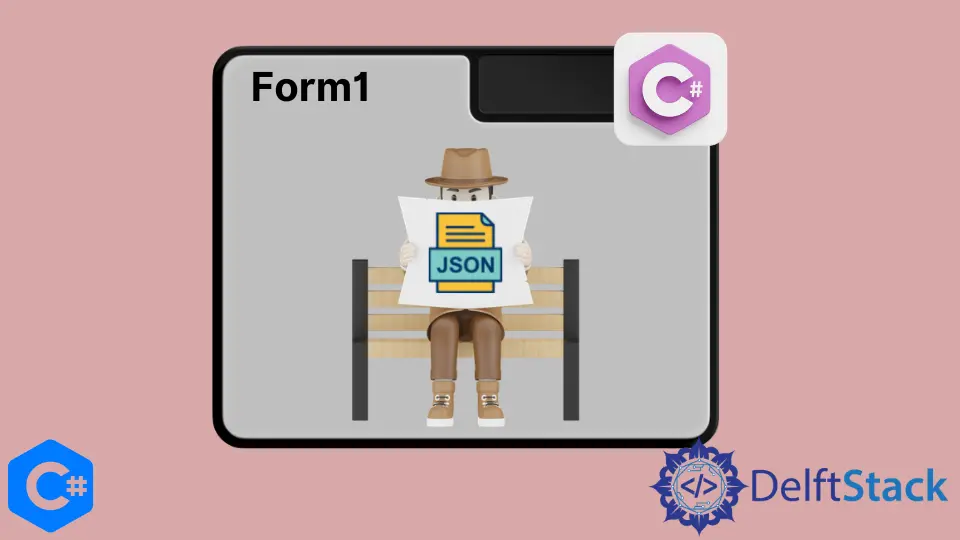
This tutorial will discuss methods to read a JSON file in C#.
Read a JSON File With the JsonConvert
Class in C#
The JsonConvert
class can be used to read JSON data into objects and variables and write objects and variables in JSON format in C#. The JsonConvert
class is present in the Json.net
framework, which does not come pre-installed. The command to install Json.net
is given below.
Install-Package Newtonsoft.Json
In this article, we will use the following JSON data.
{
"key1": "value1",
"key2": "value2",
"key3": "value3"
}
In order to read data from this file, we first have to create a model class that contains all the attributes that we want to read from the file.
file.json:
class datamodel {
public string key1 { get; set; }
public string key2 { get; set; }
public string key3 { get; set; }
}
Now we have a model class that will store all the data from the JSON file into class objects. We can get data from a file with the JsonConvert.DeserializeObject()
function in C#. The JsonConvert.DeserializeObject()
function takes the JSON data in the form of a string variable and converts it into the specified data-model class object. The following code example shows us how we can get the data from a JSON file in class objects with the JsonConvert.DeserializeObject()
function in C#.
StreamReader r = new StreamReader("file.json");
string jsonString = r.ReadToEnd();
datamodel m = JsonConvert.DeserializeObject<datamodel>(jsonString);
We initialized a StreamReader
- r
to read the file containing the JSON data file.json
. We then initialized the jsonString
, which is all the data inside the file.json
file. We created an instance of the datamodel
class m
to store the value returned by the JsonConvert.DeserializeObject<datamodel>(jsonString)
function. This the easiest method to read data from and write data to a JSON file in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn