How to Read a File to String in C#
-
Read a File to String With the
File.ReadAllText()
Method inC#
-
Read a File to String With the
StreamReader.ReadToEnd()
Method inC#
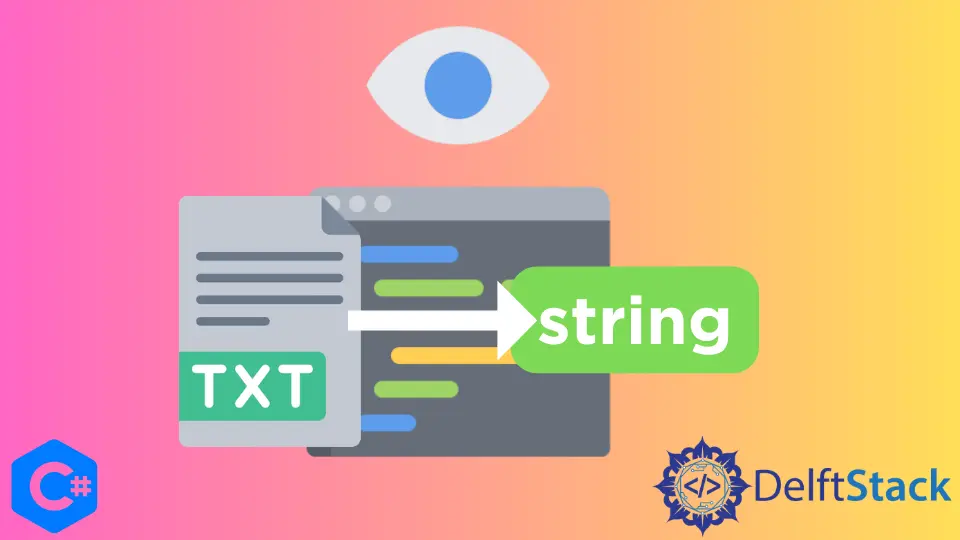
This tutorial will discuss the methods to read all the contents of a file to a string variable in C#.
Read a File to String With the File.ReadAllText()
Method in C#
The File
class provides many functions to interact with the files in C#. The [File.ReadAllText()
method](File.ReadAllText Method (System.IO) | Microsoft Docs) in C# reads all the contents of a file. The File.ReadAllText()
method takes the file’s path as an argument and returns the contents of the specified file in a string variable. See the following example code.
using System;
using System.IO;
namespace read_file_to_string {
class Program {
static void Main(string[] args) {
string text = File.ReadAllText(@"C:\File\file.txt");
Console.WriteLine(text);
}
}
}
Output:
this is all the text in this file
In the above code, we read all the contents of the file file.txt
inside the path C:\File\
into the string variable text
with the File.ReadAllText()
method in C#.
Read a File to String With the StreamReader.ReadToEnd()
Method in C#
The StreamReader
class reads the content from a byte stream with a particular encoding in C#. The StreamReader.ReadToEnd()
method is used to read all the contents of a file in C#. The StreamReader.ReadToEnd()
method returns the contents of the specified file in a string variable. See the following example code.
using System;
using System.IO;
namespace read_file_to_string {
class Program {
static void Main(string[] args) {
StreamReader fileReader = new StreamReader(@"C:\File\file.txt");
string text = fileReader.ReadToEnd();
Console.WriteLine(text);
}
}
}
Output:
this is all the text in this file
In the above code, we read all the contents of the file file.txt
inside the path C:\File\
into the string variable text
with the StreamReader.ReadToEnd()
method in C#. This approach is much faster than the previous approach.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp File
- How to Get File Name From the Path in C#
- How to Rename a File in C#
- How to Download a File From a URL in C#
- How to Read a Text File Line by Line in C#
- How to Read PDF File in C#
- How to Read Embedded Resource Text File in C#