How to Do Percentage Calculation in C#
- Understanding Percentage Calculations
- Basic Percentage Calculation
- Percentage Increase Calculation
- Percentage Decrease Calculation
- Conclusion
- FAQ
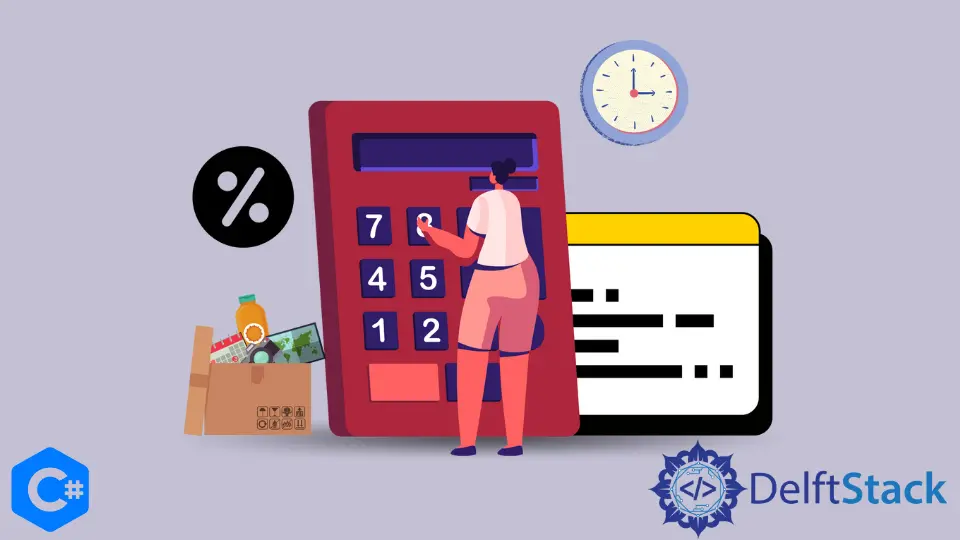
Calculating percentages is a fundamental skill in programming, especially when dealing with data analysis, financial applications, or any scenario that requires statistical insight.
In this article, we will explore how to calculate percentages in C#. Whether you’re a beginner or an experienced developer, understanding percentage calculations can enhance your coding toolkit. We will break down the methods to perform these calculations effectively, providing clear examples along the way. By the end, you will be well-equipped to implement percentage calculations in your own C# projects.
Understanding Percentage Calculations
Before diving into the code, let’s clarify what a percentage is. A percentage represents a fraction of 100. For example, if you have a score of 75 out of 100, that’s 75%. To calculate a percentage in C#, you typically multiply a value by the percentage (expressed as a decimal) and then divide by 100. This basic formula is the foundation of all percentage calculations.
Basic Percentage Calculation
The simplest way to calculate a percentage in C# is by using a straightforward formula. Here’s how you can do it:
using System;
class Program
{
static void Main()
{
double total = 200;
double percentage = 15;
double result = (percentage / 100) * total;
Console.WriteLine("The 15% of 200 is: " + result);
}
}
Output:
The 15% of 200 is: 30
In this example, we define a total value of 200 and want to find out what 15% of that total is. We first convert the percentage into a decimal by dividing it by 100, then multiply that decimal by the total. The result is 30, indicating that 15% of 200 equals 30. This method is efficient for simple calculations and can be easily modified to accommodate different totals or percentages.
Percentage Increase Calculation
Calculating a percentage increase is slightly more complex but follows a similar logic. Let’s say you want to determine how much a value increases after a certain percentage. Here’s how to do it in C#:
using System;
class Program
{
static void Main()
{
double originalValue = 100;
double increasePercentage = 20;
double increaseAmount = (increasePercentage / 100) * originalValue;
double newValue = originalValue + increaseAmount;
Console.WriteLine("The new value after a 20% increase is: " + newValue);
}
}
Output:
The new value after a 20% increase is: 120
In this code snippet, we start with an original value of 100 and want to calculate a 20% increase. We first find the increase amount by applying the percentage to the original value, then add it back to the original value to find the new total. This method is particularly useful in financial applications, where understanding growth is essential.
Percentage Decrease Calculation
Conversely, you may need to calculate a percentage decrease. This is equally important in various applications, such as discount calculations. Here’s how to perform a percentage decrease calculation in C#:
using System;
class Program
{
static void Main()
{
double originalPrice = 150;
double discountPercentage = 10;
double discountAmount = (discountPercentage / 100) * originalPrice;
double finalPrice = originalPrice - discountAmount;
Console.WriteLine("The final price after a 10% discount is: " + finalPrice);
}
}
Output:
The final price after a 10% discount is: 135
In this example, we start with an original price of 150 and apply a 10% discount. Similar to the previous method, we calculate the discount amount and subtract it from the original price to determine the final price. This approach is widely used in e-commerce applications, where discounts and prices are frequently adjusted.
Conclusion
Calculating percentages in C# is a straightforward process that can be applied in various scenarios, from simple calculations to more complex financial assessments. We covered the basics of percentage calculations, including how to calculate increases and decreases effectively. By mastering these techniques, you can enhance your programming skills and apply them to real-world problems. Remember, practice makes perfect, so don’t hesitate to experiment with different values and scenarios in your own C# projects.
FAQ
-
How do I calculate a percentage in C#?
You calculate a percentage by multiplying the percentage value (as a decimal) by the total value. -
What is the formula for percentage increase?
The formula for percentage increase is: New Value = Original Value + (Percentage Increase / 100) * Original Value. -
How do I calculate a percentage decrease in C#?
To calculate a percentage decrease, subtract the discount amount from the original value: Final Price = Original Price - (Discount Percentage / 100) * Original Price. -
Can I use these calculations for any type of data?
Yes, these percentage calculations can be applied to various types of numerical data, such as financial figures, scores, or any measurable quantity. -
What are some practical applications of percentage calculations?
Percentage calculations are commonly used in finance, sales, statistics, and data analysis, among other fields.
#. We cover basic percentage calculations, percentage increases, and decreases with clear examples. Enhance your programming skills and apply these techniques in various applications. Perfect for beginners and experienced developers alike.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn