How to Pass a Function as a Parameter in C#
-
Pass a Function as a Parameter With the
Func<>
Delegate inC#
-
Pass a Function as a Parameter Inside Another Function With the
Action<>
Delegate inC#
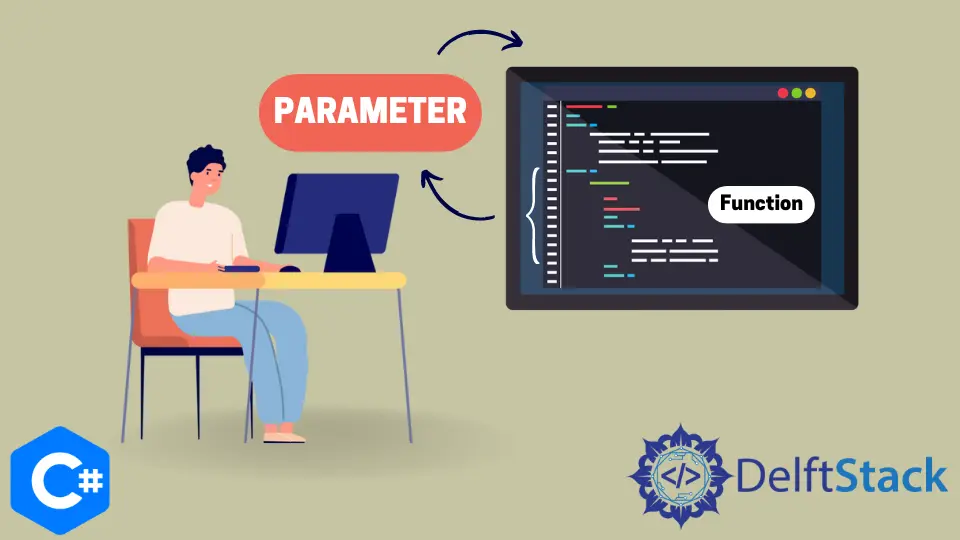
This tutorial will introduce methods to pass a function as a parameter inside another function in C#.
Pass a Function as a Parameter With the Func<>
Delegate in C#
The Func<T1, T-return>
delegate defines a function with T1
parameter and T-return
return type in C#. We can pass a function as a parameter inside another function with the Func<T1, t-return>
delegate. The following code example shows us how we can pass a function as a parameter inside another function with the Func<>
delegate in C#.
using System;
namespace pass_function_as_parameter {
class Program {
static int functionToPass(int x) {
return x + 10;
}
static void function(Func<int, int> functionToPass) {
int i = functionToPass(22);
Console.WriteLine("i = {0}", i);
}
static void Main(string[] args) {
function(functionToPass);
}
}
}
Output:
i = 32
We defined the function functionToPass(int x)
which takes an integer value as a parameter, increments it with 10
and returns the result as an integer value. We passed the functionToPass()
function as a parameter to the function()
function with the Func<int, int>
delegate. We passed the value 22
to the functionToPass()
function inside the function()
function. In the main function, we called the function with function(functionToPass)
function call. The Func<>
delegate can only be used to pass the functions that return some value.
Pass a Function as a Parameter Inside Another Function With the Action<>
Delegate in C#
If we want to pass a function that does not return a value, we have to use the Action<>
delegate in C#. The Action<T>
delegate works just like the function delegate; it is used to define a function with the T
parameter. We can use the Action<>
delegate to pass a function as a parameter to another function. The following code example shows us how we can pass a function as a parameter inside another function with the Action<>
delegate in C#.
using System;
namespace pass_function_as_parameter {
class Program {
static void functionToPass2(int x) {
int increment = x + 10;
Console.WriteLine("increment = {0}", increment);
}
static void function2(Action<int> functionToPass2) {
functionToPass2(22);
}
static void Main(string[] args) {
function2(functionToPass2);
}
}
}
Output:
increment = 32
We defined the function functionToPass2(int x)
which takes an integer value as a parameter, increments it with 10
and prints the result. We passed the functionToPass2()
function as a parameter to the function()
function with the Action<int>
delegate. We passed the value 22
to the functionToPass2()
function inside the function2()
function. In the main function, we called the function with function2(functionToPass2)
function call. The Action<>
delegate can only be used to pass the functions that do not return any value.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn