Optional Parameter in C#
-
Use the Default Value Method to Make Method Parameter Optional in
C#
-
Use the Overloading Method to Make Method Parameter Optional in
C#
-
Use the
Optional
Attribute to Make Method Parameter Optional inC#
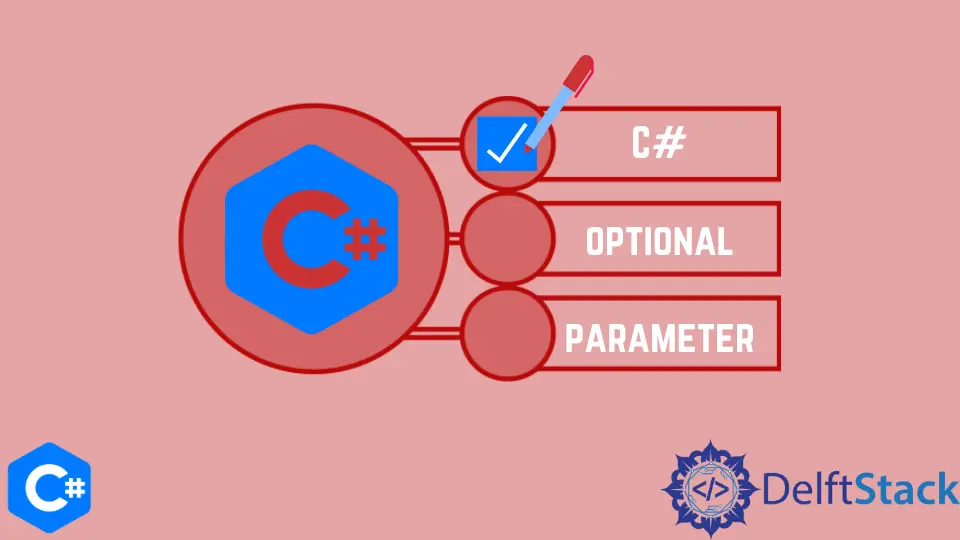
This article will introduce different methods to make the method parameter optional in C#.
Use the Default Value Method to Make Method Parameter Optional in C#
We have a user-defined function adding()
. It has two mandatory parameters, and the third parameter is optional. We will make the third parameter optional by assigning a default value to it. In that case, if we will not pass any third argument, then the function will use the default value, and the third parameter will be considered optional.
The program below shows how we can use the default value method to make the method parameter optional.
using System;
class Add {
static public void adding(int num1, int num2, int num3 = 0) {
Console.WriteLine(num1 + num2 + num3);
}
static public void Main() {
adding(4, 3);
adding(4, 3, 6);
}
}
Output:
7
13
We have called the function twice. First by passing the mandatory parameters only. Second by passing the optional parameter only.
Use the Overloading Method to Make Method Parameter Optional in C#
The other method is to create overloads of a function to make the method parameter optional. In method overloading, we create multiple methods with the same name. In this way, we can make a parameter optional.
The program below shows how we can use method overloading to make the method parameter optional.
using System;
class Add {
static public void adding(int num1, int num2) {
Console.WriteLine(num1 + num2);
}
static public void adding(int num1, int num2, int num3) {
Console.WriteLine(num1 + num2 + num3);
}
static public void Main() {
adding(4, 3);
adding(4, 3, 6);
}
}
Output:
7
13
Use the Optional
Attribute to Make Method Parameter Optional in C#
Another method is to use the Optional
keyword enclosed in square brackets before the parameter name to make the method parameter optional.
The program below shows how we can use the Optional attribute to make the method parameter optional.
using System;
using System.Runtime.InteropServices;
class Add {
static public void adding(int num1, int num2, [Optional] int num3) {
Console.WriteLine(num1 + num2 + num3);
}
static public void Main() {
adding(4, 3);
adding(4, 3, 6);
}
}
Output:
7
13
Related Article - Csharp Method
- How to Pass a Method as a Parameter in C# Function
- How to Sort a List by a Property in the Object in C#
- How to Pass Multiple Parameters to a Get Method in C#
- How to Override a Static Method in C#
- Method Group in C#