How to Add Newline to String in C#
-
Add a New Line to a String With the
\n
Escape Character inC#
-
Add a New Line to a String With the
Environment.NewLine
Property inC#
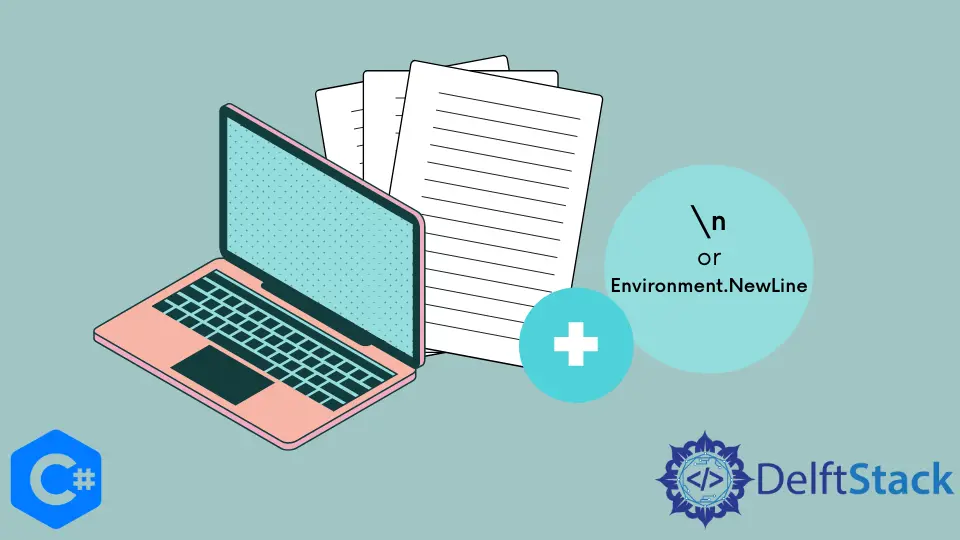
This tutorial will introduce methods to add a new line to a string variable in C#.
Add a New Line to a String With the \n
Escape Character in C#
The \n
or the \r
escape character in Mac is used to add a new line to the console in C#. For a Windows machine, we should use the \n
escape character for line breaks. The line break can also be used to add multiple lines to a string variable. We have to write \n
in the string wherever we want to start a new line. The following code example shows us how to add a new line to a string variable with the \n
escape character in C#.
using System;
namespace add_newline_to_string {
class Program {
static void Main(string[] args) {
string s = "This is the first line.\nThis is the second line.";
Console.WriteLine(s);
}
}
}
Output:
This is the first line.
This is the second line.
We added a new line to the string variable s
with the \n
escape character in C#. The only drawback of this method is that we have to write \n
during the initialization of the string variable s
. The above code can be modified to add a line break to the string variable s
after the initialization with the String.Replace()
function. The String.Replace(string x, y)
returns a string in which the string x
is replaced with the string y
. The following code example shows us how we can add \n
after initialization with the String.Replace()
function in C#.
using System;
namespace add_newline_to_string {
class Program {
static void Main(string[] args) {
string s = "This is the first line.This is the second line.";
s = s.Replace(".", ".\n");
Console.WriteLine(s);
}
}
}
Output:
This is the first line.
This is the second line.
We added a new line to the string variable s
after initialization with the String.Replace()
function in C#. This method of adding a new line to a string is not optimal because \n
escape character is environment-dependent. We need to know the environment where our code is running to correctly add a new line to a string variable with this approach.
Add a New Line to a String With the Environment.NewLine
Property in C#
If we want to add a new line to our code, but we have no idea about the environment in which our code will be running, we can use the Environment.NewLine
property in C#. The Environment.NewLine
property gets the appropriate newline for our environment. The following code example shows us how to add a new line to a string with the Environment.NewLine
property in C#.
using System;
namespace add_newline_to_string {
class Program {
static void Main(string[] args) {
string s = "This is the first line.This is the second line.";
s = s.Replace(".", "." + Environment.NewLine);
Console.WriteLine(s);
}
}
}
Output:
This is the first line.
This is the second line.
We initialized a string variable s
and added a new line to the string variable s
after initialization with the Environment.NewLine
property and the String.Replace()
function in C#. This method is preferable over the other because, in this method, we don’t have to worry about the environment in which our code will be executing.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn