Network Programming in C#
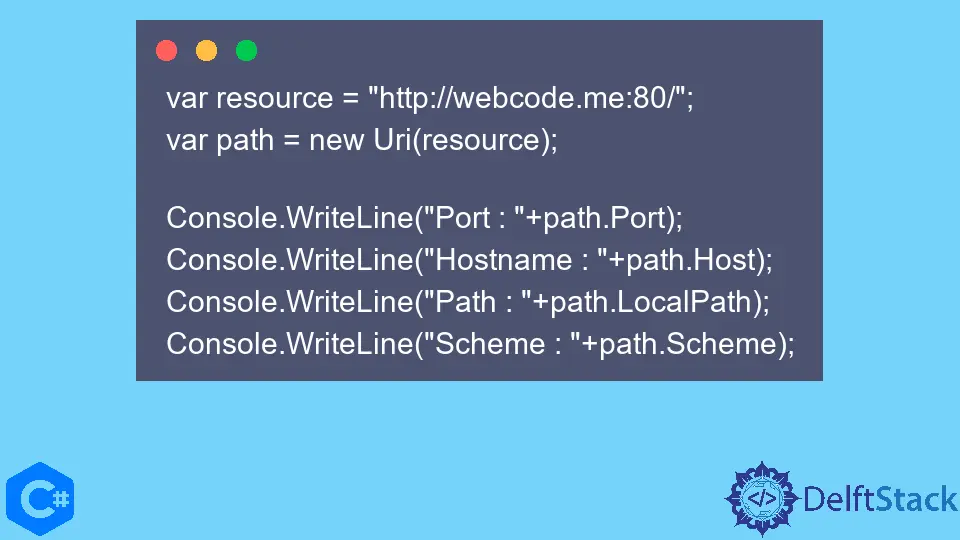
This tutorial demonstrates how we can use the System.NET
library provided by the .NET framework to create a network application in C#.
Network Programming in C#
The System.NET
library of the .NET framework provides a simple framework for network programming in C#.
There are two kinds of network applications; client and server. The client applications request the server application to perform a task over the network, and the server application performs that task and returns the results to the client application.
We can program this client-server application with the System.NET
namespace in C#. Let’s start with learning URI
below.
Uniform Resource Indicator
In networking, the client uses a uniform resource indicator or URI
to specify the type of resource required. The URI
is made up of different fragments:
scheme identifier
- It identifies the communications protocol for the request and response.server identifier
- A DNS hostname or a TCP address uniquely identifies the server on the Internet.path identifier
- It locates the information that we requested on the server; and an optional query string, which passes information from the client to the server.
The URI
class in the System.NET
namespace creates a uniform resource identifier in C#. The following code block shows us how to use the URI
class in C#.
var resource = "http://webcode.me:80/";
var path = new Uri(resource);
Console.WriteLine("Port : " + path.Port);
Console.WriteLine("Hostname : " + path.Host);
Console.WriteLine("Path : " + path.LocalPath);
Console.WriteLine("Scheme : " + path.Scheme);
Output:
Port: 80
Hostname: webcode.me
Path: /
Scheme: http
The above code takes a string
, converts that string
into a URI
, and then prints different fragments of that newly created URI
. In addition, this URI
class automatically performs some validations to ensure that the URIs
are well-formatted.
These validations can sometimes backfire and break the whole URI
. We can also disable this effect by setting UriCreationOptions.DangerousDisablePathAndQueryCanonicalization
to true
.
It disables the validations, so we cannot use the Uri.Fragments
property.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn