Message Box in C#
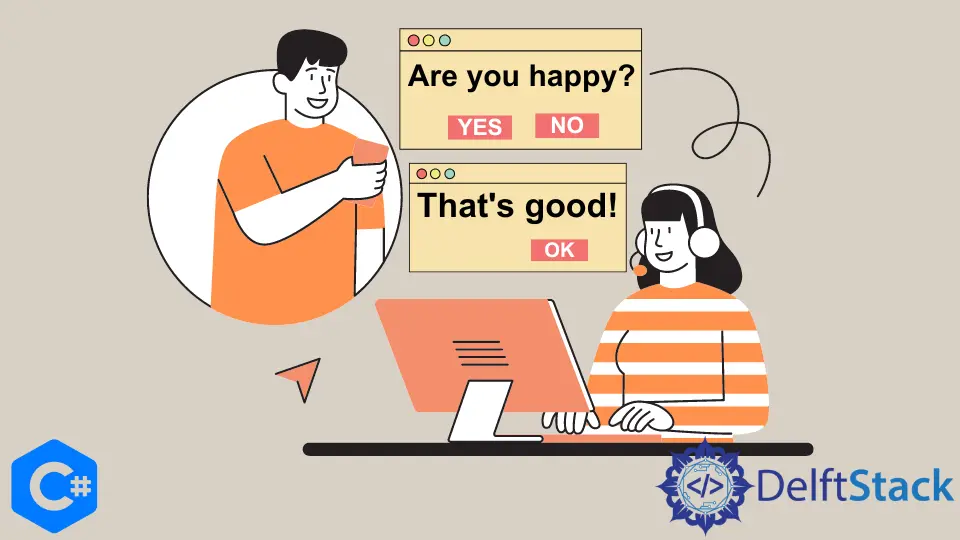
This tutorial will discuss how to create a message box with yes
or no
options in C#.
Create a Message Box With the MessageBox
Class in C#
The MessageBox
class displays a message window in C#. If we want to create a message box with yes
and no
options, we can pass the MessageBoxButton.YesNo
enum in the parameters of the MessageBox
class constructor. The following code example shows us how we can create a message box with yes
and no
options with the MessageBox
class in C#.
using System.Windows.Forms;
namespace messagbox {
static class Program {
static void Main() {
Application.EnableVisualStyles();
DialogResult dr = MessageBox.Show("Are you happy now?", "Mood Test", MessageBoxButtons.YesNo);
switch (dr) {
case DialogResult.Yes:
MessageBox.Show("That is Fantastic");
break;
case DialogResult.No:
MessageBox.Show("Why Not?");
break;
}
}
}
}
Output:
In the above code, we stored the results of the MessageBox.Show()
function inside the dr
instance of the DialogResult
enum. The DialogResult
enum contains identifiers to represent the values returned by a dialog box. We used a switch
statement to check the value returned by the MessageBox.Show()
function and displayed another message box for each selected option.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn