How to IIF Equivalent in C#
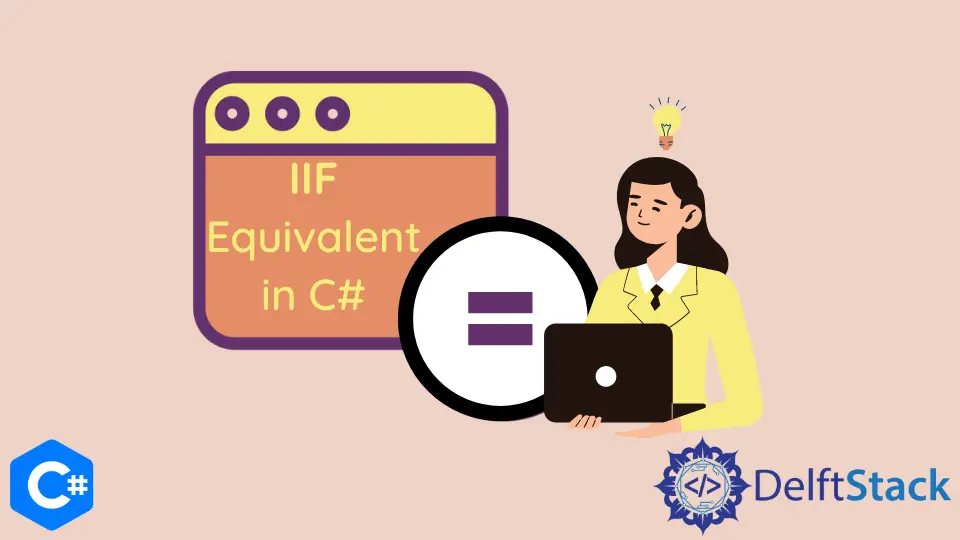
This tutorial will demonstrate how to use the IIF Equivalent in C# and other alternatives.
IIF
stands for immediate if
and is available in the Visual Basic programming language. In a single line, you can pass a particular condition and specify the values to be returned if the condition is found to be true or false.
IIF(condition, True, False)
While C# does not have an exact equivalent to this, you can use the ?
operator.
C# ?
Operator
A ternary operator, also commonly known as a conditional operator, is an operator that accepts three operands rather than the usual one or two, which most operators use. In this case, the ?
operator simplifies an if-else block. The general structure of using the ?
operator is as follows:
string string_val = (anyBool ? "Value if True" : "Value if False");
Example:
using System;
using System.Collections.Generic;
namespace StringJoin_Example {
class Program {
static void Main(string[] args) {
// Initialize the integer variable a
int a = 1;
// Check if the condition is true
string ternary_value = (a == 1 ? "A is Equal to One" : "A is NOT Equal to One");
// Print the result to the console
Console.WriteLine("Initial Value (" + a.ToString() + "): " + ternary_value);
// Update the variable
a = 2;
// Re check if the conidition is still true
ternary_value = (a == 1 ? "A is Equal to One" : "A is NOT Equal to One");
// Print the result to the console
Console.WriteLine("Updated Value (" + a.ToString() + "): " + ternary_value);
Console.ReadLine();
}
}
}
In the example above, we created an integer variable as well as a string whose value changes depending on the value of the integer a
. To set the string value, we check the value of a
using the ternary operator. If a
is found to be equal to one, the string will reflect that and vice versa. After changing the value of a
, you can observe that the string value changes as expected in the output printed.
Output:
Initial Value (1): A is Equal to One
Updated Value (2): A is NOT Equal to One