How to Write a Multiline String Literal in C#
Minahil Noor
Feb 02, 2024
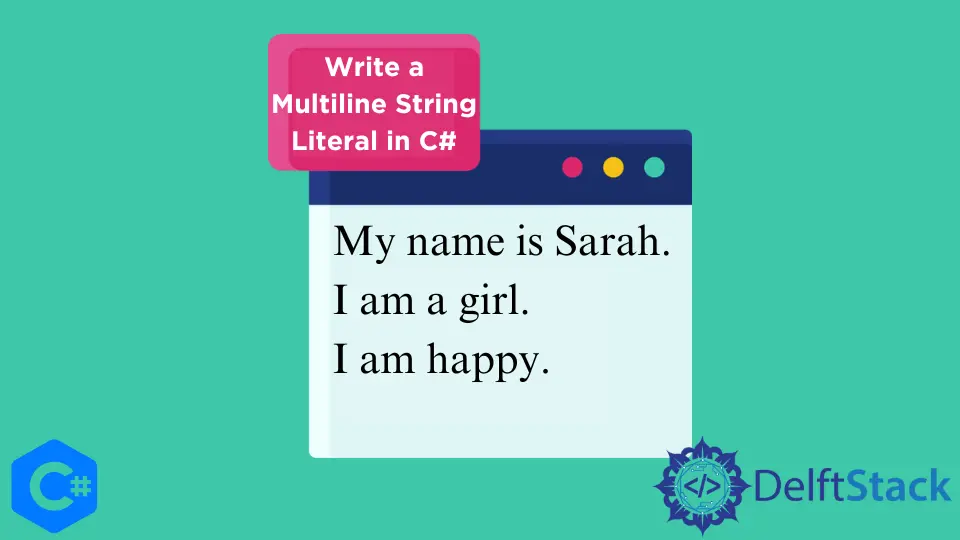
This article will introduce a method to write a multiline string literal in C#.
- Using the verbatim symbol
Use the verbatim symbol to write a multiline string literal in C#
In C#, we can use the verbatim symbol to create a verbatim string. A verbatim string is a string that contains multiple lines. This symbol is @
. The correct syntax to use this symbol is as follows
string verbatim = @" ";
The program below shows how we can use the @
symbol to write a multiline string.
using System;
using System.IO;
class MultilineString {
static void Main() {
string multiline =
@"My name is Sarah.
I am a girl.
I am happy.";
Console.WriteLine(multiline);
}
}
Output:
My name is Sarah.
I am a girl.
I am happy.