How to Round a Decimal Value to 2 Decimal Places C#
-
C# Program to Round a
Decimal
Value to 2Decimal
Places Usingdecimal.Round()
Method -
C# Program to Round a
Decimal
Value to 2Decimal
Places UsingMath.Round()
Method
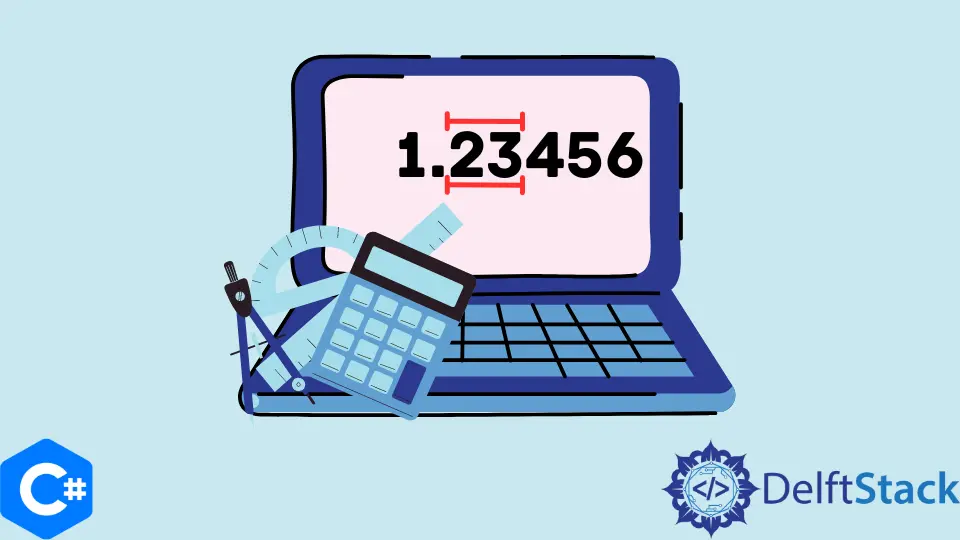
In C#, we can easily round off a decimal
number using different methods, for example, decimal.Round()
and Math.Round()
.
This article will focus on the methods to round a floating value to 2 decimal places.
C# Program to Round a Decimal
Value to 2 Decimal
Places Using decimal.Round()
Method
The method decimal.Round()
is the simplest method that is used to round off a decimal
number to a specified number of digits. This method allows up to 28 decimal
places.
The correct syntax to use this method is as follows:
decimal.Round(decimalValueName, integerValue);
Example Code:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 123.456M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
Output:
The Decimal Value Before Applying Method: 123.456
The Decimal Value After Applying Method: 123.46
This method throws ArgumentOutOfRangeException
if the integer
value that tells the number of decimal
places to round is not in a range of 0-28. This exception
is then handled by using a try-catch
block.
There is another way to use decimal.Round()
method, example code is given below:
Example Code:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 12.345M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2, MidpointRounding.AwayFromZero);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
Output:
The Decimal Value Before Applying Method: 12.345
The Decimal Value After Applying Method: 12.35
MidpointRounding.AwayFromZero
is used to round the number away from zero. Its counterpart is MidpointRounding.ToEven
, which rounds the given decimal number towards its nearest even number.
Example Code:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 12.345M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2, MidpointRounding.ToEven);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
Output:
The Decimal Value Before Applying Method: 12.345
The Decimal Value After Applying Method: 12.34
C# Program to Round a Decimal
Value to 2 Decimal
Places Using Math.Round()
Method
The method Math.Round()
is the same as the decimal.Round()
method in its functionality.
The correct syntax to use this method is as follows:
Math.Round(decimalValueName, integerValue);
Example Code:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 123.456M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = Math.Round(decimalValue, 2);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
Output:
The Decimal Value Before Applying Method: 123.456
The Decimal Value After Applying Method: 123.46
It also throws exceptions
just like the decimal.Round()
method, which is then handled using the try-catch
block. We could also specify the MidpointRounding
method as that in decimal.Round()
method.