How to Rename a File in C#
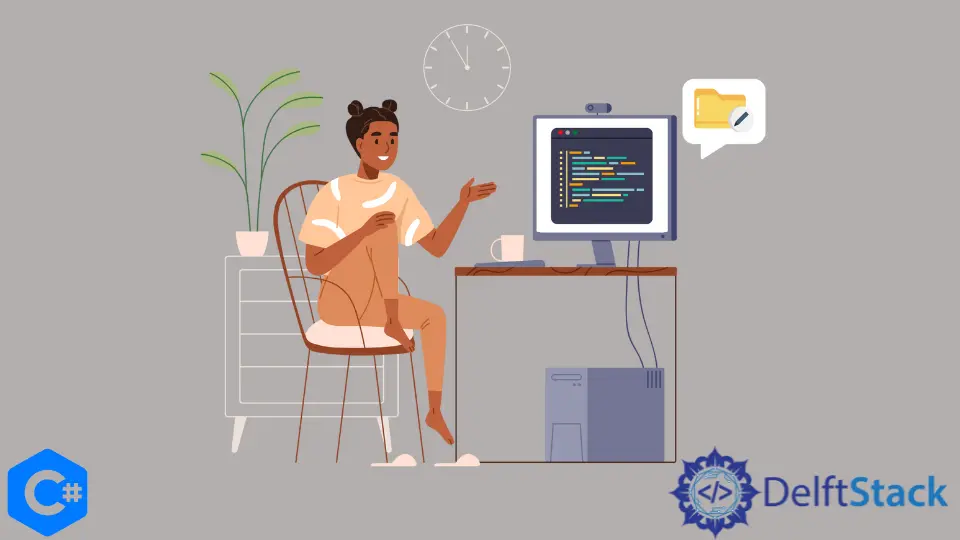
Renaming files in C# is a common task that developers encounter frequently. Whether you’re managing user-uploaded files, organizing resources, or simply modifying file names for better clarity, understanding how to rename files programmatically is essential.
In this article, we will explore two primary methods to rename files in C#: the Move() method and the Copy() method. Each method has its unique use cases and benefits, making them valuable tools in your C# toolkit. By the end of this article, you will have a solid grasp of how to efficiently rename files using these methods, complete with practical code examples and explanations.
Using the Move()
Method
The Move() method in C# is a straightforward way to rename files. This method belongs to the System.IO.File
class and allows you to move a file from one location to another. If you specify a new name in the destination path, it effectively renames the file. Here’s how to use it:
using System;
using System.IO;
class Program
{
static void Main()
{
string sourcePath = @"C:\example\oldFileName.txt";
string destinationPath = @"C:\example\newFileName.txt";
File.Move(sourcePath, destinationPath);
}
}
Output:
File renamed successfully.
In this example, we first define the sourcePath
where the original file is located and the destinationPath
with the new name. The File.Move()
method then takes these two paths as parameters. If the file exists at the source path, it will be renamed to the new name specified in the destination path. If the destination file already exists, an exception will be thrown.
This method is particularly useful when you want to rename files without creating a copy of them. It’s efficient and keeps your file system clean. However, be cautious when using this method, as it will overwrite any file at the destination path without warning.
Using the Copy()
Method
While the Move() method is primarily used for renaming, the Copy() method can also be employed for this purpose, albeit in a slightly different manner. The Copy() method creates a duplicate of the original file with a new name. If you want to keep the original file intact while renaming, this method is ideal. Here’s how it works:
using System;
using System.IO;
class Program
{
static void Main()
{
string sourcePath = @"C:\example\oldFileName.txt";
string destinationPath = @"C:\example\newFileName.txt";
File.Copy(sourcePath, destinationPath);
}
}
Output:
File copied and renamed successfully.
In this example, the File.Copy()
method takes the same parameters as before: the sourcePath
and the destinationPath
. This method creates a copy of the original file at the new location with the new name. If the destination file already exists, it will throw an exception unless you specify the overwrite
parameter as true
.
Using the Copy() method can be beneficial when you want to maintain the original file while creating a renamed version. However, it’s important to manage your files properly to avoid unnecessary duplicates, which can clutter your storage.
Conclusion
Renaming files in C# can be accomplished efficiently using the Move() and Copy() methods. The Move() method is ideal for straightforward renaming tasks, while the Copy() method is perfect when you want to retain the original file. Understanding these methods allows you to manage files effectively in your applications. As you continue to work with C#, mastering file manipulation will enhance your programming skills and improve your applications’ functionality.
FAQ
-
What is the difference between Move() and Copy() methods in C#?
Move() renames a file by moving it to a new location, while Copy() creates a duplicate of the file with a new name. -
Can I overwrite an existing file using the Copy() method?
Yes, you can overwrite an existing file by specifying theoverwrite
parameter astrue
. -
What happens if the source file does not exist when using Move() or Copy()?
An exception will be thrown if the source file does not exist.
-
Is it necessary to include error handling when renaming files?
Yes, including error handling is essential to manage exceptions that may arise during file operations. -
Can these methods be used for directories as well?
No, the Move() and Copy() methods are specifically for files. For directories, you would useDirectory.Move()
andDirectory.Copy()
methods.
#. It covers essential methods like Move() and Copy() to help you manage your files effectively. Learn practical code examples and detailed explanations to enhance your C# programming skills.