How to Read and Parse an XML File in C#
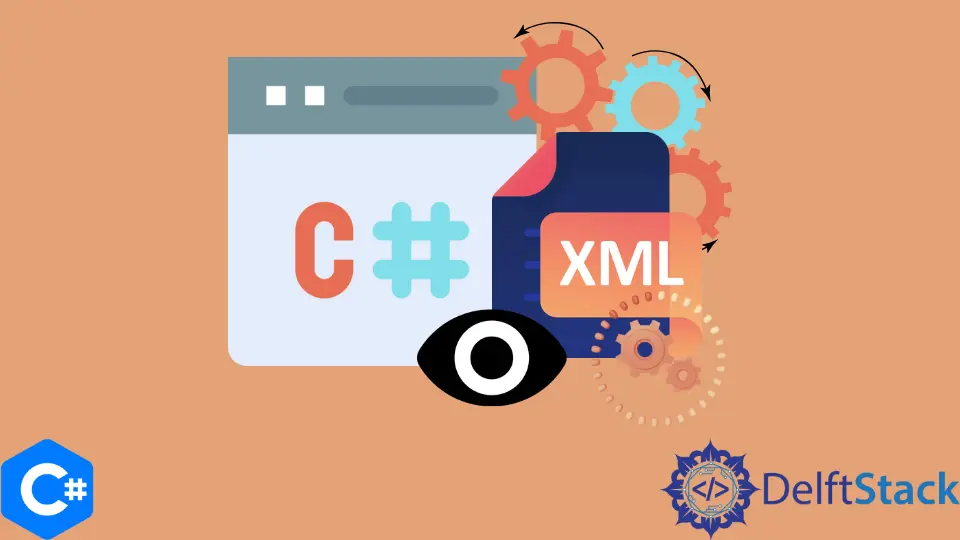
In C#, System.Xml
namespace is used to deal with the XML
files. It has different classes and methods to process XML
files. We can read, parse and write an XML
file using this namespace.
In this article, we are going to discuss different methods that are used to read and parse an XML
file.
C# Program to Read and Parse an XML
File Using XmlReader
Class
The XmlReader
class in C# provides an efficient way to access XML
data. XmlReader.Read()
method reads the first node of the XML
file and then reads the whole file using a while
loop.
The correct syntax to use this method is as follows:
XmlReader VariableName = XmlReader.Create(@"filepath");
while (reader.Read()) {
// Action to perform repeatidly
}
The XML
file that we have read and parsed in our program is as follows. Copy and paste this code into a new text file and save it as .xml
to use this file for executing the program given below.
<?xml version="1.0" encoding="utf-8"?>
<Students>
<Student>
</Student>
<Student>
<Name>Olivia</Name>
<Grade>A</Grade>
</Student>
<Student>
<Name>Laura</Name>
<Grade>A+</Grade>
</Student>
<Student>
<Name>Ben</Name>
<Grade>A-</Grade>
</Student>
<Student>
<Name>Henry</Name>
<Grade>B</Grade>
</Student>
<Student>
<Name>Monica</Name>
<Grade>B</Grade>
</Student>
</Students>
Example Code:
using System;
using System.Xml;
namespace XMLReadAndParse {
class XMLReadandParse {
static void Main(string[] args) {
// Start with XmlReader object
// here, we try to setup Stream between the XML file nad xmlReader
using (XmlReader reader = XmlReader.Create(@"d:\Example.xml")) {
while (reader.Read()) {
if (reader.IsStartElement()) {
// return only when you have START tag
switch (reader.Name.ToString()) {
case "Name":
Console.WriteLine("The Name of the Student is " + reader.ReadString());
break;
case "Grade":
Console.WriteLine("The Grade of the Student is " + reader.ReadString());
break;
}
}
Console.WriteLine("");
}
}
Console.ReadKey();
}
}
}
Here we have created an object of XmlReader
and then created a reader stream of the given XML
file using Create()
method.
Then, we have used XmlReader.Read()
method to read the XML
file. This method returns a bool
value that indicates whether our created stream contains the XML
statements or not.
After that, we have checked if there is any starting element using XmlReader.IsStartElement()
method. Since we have two element fields in our XML
data, we have used the switch
statement to read the data from both the fields using the ReadString()
method.
Output:
The Name of the Student is Olivia
The Grade of the Student is A
The Name of the Student is Laura
The Grade of the Student is A+
The Name of the Student is Ben
The Grade of the Student is A-
The Name of the Student is Henry
The Grade of the Student is B
The Name of the Student is Monica
The Grade of the Student is B