How to Download a File From a URL in C#
- Using the WebClient Class
- Using HttpClient for File Downloads
- Best Practices for Downloading Files
- Conclusion
- FAQ
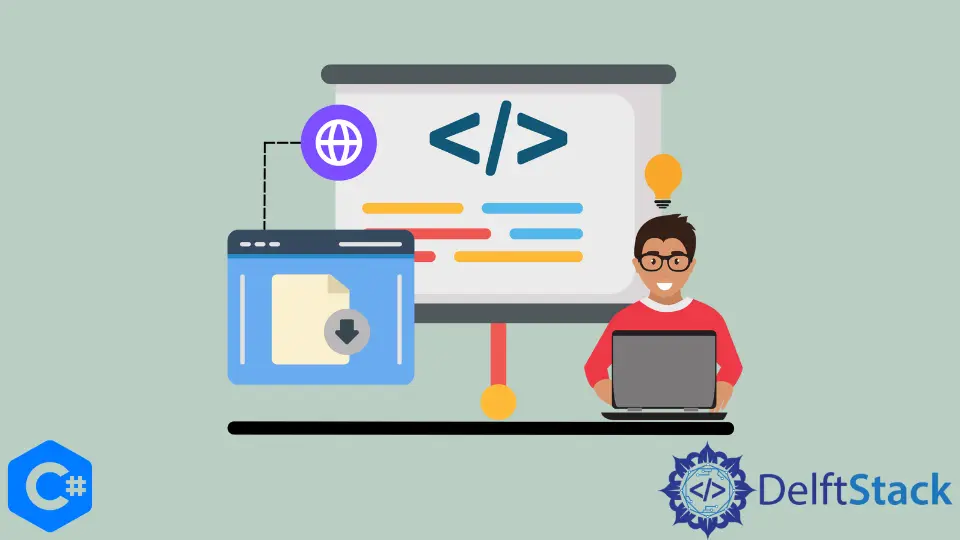
Downloading files from a URL in C# can be a straightforward task, especially with the right methods and libraries at your disposal. Whether you’re building a web application or a desktop application, being able to download files programmatically is a valuable skill.
In this article, we will explore how to use the DownloadFile()
method from the WebClient class, which simplifies the process of downloading files from the internet. We will also discuss some best practices and offer clear code examples to ensure you can implement this functionality seamlessly. Let’s dive into the world of file downloading in C#!
Using the WebClient Class
The WebClient class provides a simple way to download files from a URL. It is part of the System.Net namespace and offers methods that enable you to send data to and receive data from a resource identified by a URI. The DownloadFile()
method is particularly useful for downloading files with minimal code.
Here’s a basic example of how to use the WebClient class to download a file:
using System;
using System.Net;
class Program
{
static void Main()
{
string url = "https://example.com/file.zip";
string destinationPath = @"C:\Downloads\file.zip";
using (WebClient client = new WebClient())
{
client.DownloadFile(url, destinationPath);
}
Console.WriteLine("File downloaded successfully!");
}
}
In this example, we first create an instance of the WebClient class. We specify the URL of the file we want to download and the destination path where we want to save the file. The DownloadFile()
method is then called, which takes the URL and the destination path as parameters. Once the download is complete, a success message is printed to the console.
Output:
File downloaded successfully!
The WebClient class handles the underlying complexities of web requests, making it an excellent choice for simple file downloads. However, it is important to note that the WebClient class is considered somewhat outdated, and for more robust applications, you might want to consider using HttpClient instead.
Using HttpClient for File Downloads
The HttpClient class is part of the System.Net.Http namespace and provides a more modern approach to making HTTP requests. It is more flexible than WebClient and is the recommended way to perform web requests in C#. Here’s how you can use HttpClient to download a file:
using System;
using System.Net.Http;
using System.IO;
using System.Threading.Tasks;
class Program
{
static async Task Main()
{
string url = "https://example.com/file.zip";
string destinationPath = @"C:\Downloads\file.zip";
using (HttpClient client = new HttpClient())
{
var response = await client.GetAsync(url);
response.EnsureSuccessStatusCode();
using (var fs = new FileStream(destinationPath, FileMode.Create, FileAccess.Write, FileShare.None))
{
await response.Content.CopyToAsync(fs);
}
}
Console.WriteLine("File downloaded successfully!");
}
}
In this example, we utilize the asynchronous capabilities of HttpClient. We first create an instance of HttpClient and send a GET request to the specified URL. The EnsureSuccessStatusCode()
method checks if the request was successful. If so, we create a FileStream to write the response content to the specified destination path. The response content is then copied to the FileStream asynchronously, ensuring the application remains responsive during the download.
Output:
File downloaded successfully!
Using HttpClient is beneficial for larger applications due to its support for asynchronous programming and better resource management. It allows for more complex scenarios, such as handling headers, cookies, and more.
Best Practices for Downloading Files
When downloading files from a URL in C#, there are several best practices to keep in mind:
-
Error Handling: Always implement error handling to manage exceptions that may occur during the download process. This includes handling network errors, file access issues, and invalid URLs.
-
Asynchronous Programming: Utilize asynchronous methods to keep your application responsive. This is especially important in UI applications where blocking the main thread can lead to a poor user experience.
-
Resource Management: Make sure to dispose of your HttpClient and FileStream instances properly to avoid memory leaks. Using the
using
statement is a good practice here. -
Progress Reporting: If you’re downloading large files, consider implementing progress reporting to inform users about the download status. This can enhance the user experience significantly.
By following these best practices, you can ensure that your file downloading functionality is robust, efficient, and user-friendly.
Conclusion
Downloading files from a URL in C# can be accomplished easily using either the WebClient or HttpClient classes. While WebClient is simpler and easier to use for basic tasks, HttpClient provides more flexibility and is better suited for modern applications. By understanding how to implement these methods and following best practices, you can create effective file downloading functionality in your applications. Remember to always test your code thoroughly and handle exceptions appropriately for a seamless user experience.
FAQ
-
What is the difference between WebClient and HttpClient?
WebClient is simpler and easier to use for basic tasks, while HttpClient is more flexible and suitable for modern applications, particularly those that require asynchronous programming. -
Can I download files asynchronously in C#?
Yes, you can use the HttpClient class to download files asynchronously, which helps keep your application responsive during the download process. -
What should I do if the file download fails?
Implement error handling in your code to manage exceptions and provide feedback to the user. You can also log errors for further analysis. -
Is it necessary to dispose of HttpClient or WebClient?
Yes, it is important to dispose of these objects properly to free up resources and avoid memory leaks. Using theusing
statement is recommended. -
Can I download files from secure URLs (HTTPS)?
Yes, both WebClient and HttpClient support downloading files from secure URLs. Just ensure that the URL is correct and that you have the necessary permissions.