How to Create a Folder in C#
- Understanding the CreateDirectory Method
- Handling Exceptions During Folder Creation
- Creating Nested Directories
- Conclusion
- FAQ
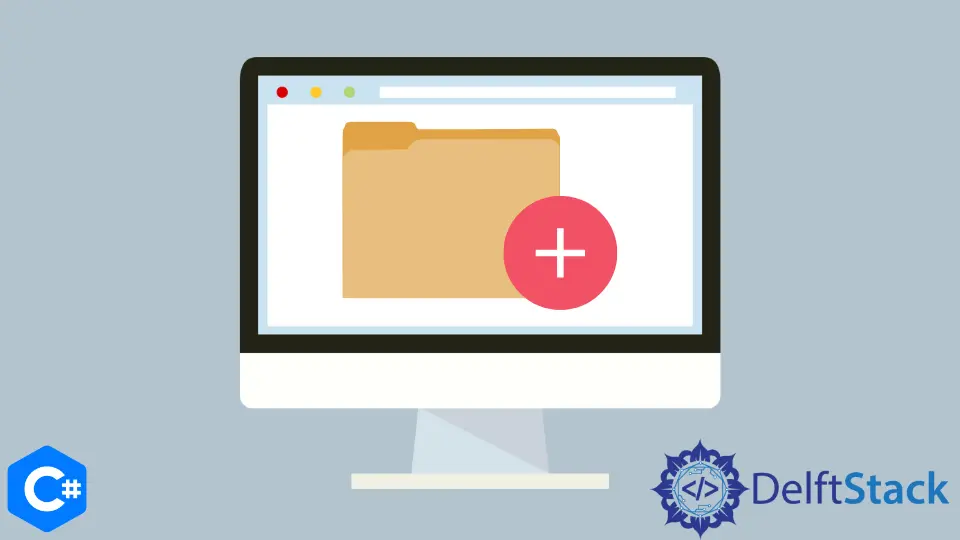
Creating a folder in C# is a straightforward task that can be accomplished using the built-in methods provided by the .NET framework. Whether you are developing a desktop application, a web service, or any other type of software, managing files and directories is often a crucial part of the process.
In this article, we will explore how to create a new folder if it does not exist, utilizing the CreateDirectory()
method from the System.IO
namespace. This method is not only efficient but also user-friendly, making it an excellent choice for developers of all skill levels. We will walk through the code step-by-step, ensuring you have a clear understanding of how to implement this functionality in your C# applications.
Understanding the CreateDirectory Method
The CreateDirectory()
method is part of the System.IO
namespace and is designed to create a directory at a specified path. If the directory already exists, this method does nothing and simply returns a DirectoryInfo
object representing the existing directory. This feature is particularly useful because it eliminates the need for additional checks to see if the folder is already present.
Here’s a simple example of using the CreateDirectory()
method:
using System;
using System.IO;
class Program
{
static void Main()
{
string folderPath = @"C:\ExampleFolder";
DirectoryInfo directoryInfo = Directory.CreateDirectory(folderPath);
Console.WriteLine($"Folder created at: {directoryInfo.FullName}");
}
}
When you run the above code, it attempts to create a folder named “ExampleFolder” at the specified path. If the folder already exists, it will simply return the existing directory’s information.
Output:
Folder created at: C:\ExampleFolder
This method is simple yet powerful. It allows developers to focus on other aspects of their applications without worrying about directory management. The Directory.CreateDirectory()
method is also thread-safe, meaning it can be safely called from multiple threads without causing issues.
Handling Exceptions During Folder Creation
While the CreateDirectory()
method is efficient, it’s essential to handle potential exceptions that may arise during folder creation. For instance, issues like insufficient permissions or invalid paths can lead to exceptions. To address this, you can wrap your folder creation logic in a try-catch block.
Here’s how you can implement error handling:
using System;
using System.IO;
class Program
{
static void Main()
{
string folderPath = @"C:\ExampleFolder";
try
{
DirectoryInfo directoryInfo = Directory.CreateDirectory(folderPath);
Console.WriteLine($"Folder created at: {directoryInfo.FullName}");
}
catch (UnauthorizedAccessException)
{
Console.WriteLine("Error: You do not have permission to create this folder.");
}
catch (IOException ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
}
In this code, we catch two specific exceptions: UnauthorizedAccessException
and IOException
. The first one handles cases where the user lacks the necessary permissions to create a folder, while the second one catches any other I/O-related issues that may arise.
Output:
Folder created at: C:\ExampleFolder
By implementing exception handling, you can provide users with informative error messages, improving the overall user experience. This practice is crucial for any production-level application, where robustness and reliability are key.
Creating Nested Directories
In some scenarios, you may need to create nested directories, meaning that you want to create multiple folders within each other. The CreateDirectory()
method handles this elegantly. If the parent directory doesn’t exist, it will create it along with the child directories.
Here’s an example of creating nested directories:
using System;
using System.IO;
class Program
{
static void Main()
{
string nestedFolderPath = @"C:\ExampleFolder\SubFolder\AnotherSubFolder";
DirectoryInfo directoryInfo = Directory.CreateDirectory(nestedFolderPath);
Console.WriteLine($"Nested folders created at: {directoryInfo.FullName}");
}
}
When you run this code, it will create a folder structure that includes “ExampleFolder”, “SubFolder”, and “AnotherSubFolder”. If any of these folders already exist, they will be ignored, and the existing structure will remain intact.
Output:
Nested folders created at: C:\ExampleFolder\SubFolder\AnotherSubFolder
This feature is particularly useful for organizing files in a structured manner. By leveraging the CreateDirectory()
method for nested folders, developers can create complex directory hierarchies with minimal effort.
Conclusion
Creating folders in C# is a simple yet essential task for many applications. Utilizing the CreateDirectory()
method allows you to create new directories efficiently and handle existing ones gracefully. By implementing error handling and supporting nested directory creation, you can ensure that your applications remain robust and user-friendly. Whether you are developing a small utility or a large-scale application, mastering folder creation in C# is a valuable skill that will enhance your programming capabilities.
FAQ
-
How do I check if a folder exists in C#?
You can use theDirectory.Exists()
method to check if a folder exists at a specified path. -
Can I create a folder in a restricted location?
If you do not have the necessary permissions, you will encounter anUnauthorizedAccessException
.
-
What happens if I try to create a folder that already exists?
TheCreateDirectory()
method will do nothing and return the existing directory’s information. -
Can I create multiple folders at once?
Yes, you can create nested folders using theCreateDirectory()
method, which will create all necessary parent directories. -
Is the
CreateDirectory()
method thread-safe?
Yes, it is thread-safe, allowing multiple threads to call it without causing issues.